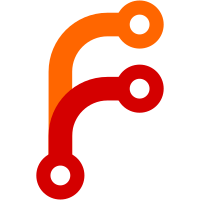
This file revealed several problems with our ASF parser: 1) The packet count in the ASF was actually a 64 bit value, leading to overflow in very long files. 2) Seeking blindly trusted the bitrate listed in the ASF header rather than computing it from the packet size and number of packets. Fix these problems and fix a few minor issues. Change-Id: Ie0f68734e6423e837757528ddb155f3bdcc979f3
49 lines
1.6 KiB
C
49 lines
1.6 KiB
C
#ifndef _ASF_H
|
|
#define _ASF_H
|
|
|
|
#include <inttypes.h>
|
|
|
|
/* ASF codec IDs */
|
|
#define ASF_CODEC_ID_WMAV1 0x160
|
|
#define ASF_CODEC_ID_WMAV2 0x161
|
|
#define ASF_CODEC_ID_WMAPRO 0x162
|
|
#define ASF_CODEC_ID_WMAVOICE 0x00A
|
|
|
|
enum asf_error_e {
|
|
ASF_ERROR_INTERNAL = -1, /* incorrect input to API calls */
|
|
ASF_ERROR_OUTOFMEM = -2, /* some malloc inside program failed */
|
|
ASF_ERROR_EOF = -3, /* unexpected end of file */
|
|
ASF_ERROR_IO = -4, /* error reading or writing to file */
|
|
ASF_ERROR_INVALID_LENGTH = -5, /* length value conflict in input data */
|
|
ASF_ERROR_INVALID_VALUE = -6, /* other value conflict in input data */
|
|
ASF_ERROR_INVALID_OBJECT = -7, /* ASF object missing or in wrong place */
|
|
ASF_ERROR_OBJECT_SIZE = -8, /* invalid ASF object size (too small) */
|
|
ASF_ERROR_SEEKABLE = -9, /* file not seekable */
|
|
ASF_ERROR_SEEK = -10, /* file is seekable but seeking failed */
|
|
ASF_ERROR_ENCRYPTED = -11 /* file is encrypted */
|
|
};
|
|
|
|
struct asf_waveformatex_s {
|
|
uint32_t packet_size;
|
|
int audiostream;
|
|
uint16_t codec_id;
|
|
uint16_t channels;
|
|
uint32_t rate;
|
|
uint32_t bitrate;
|
|
uint16_t blockalign;
|
|
uint16_t bitspersample;
|
|
uint16_t datalen;
|
|
uint64_t numpackets;
|
|
uint8_t data[46];
|
|
};
|
|
typedef struct asf_waveformatex_s asf_waveformatex_t;
|
|
|
|
int asf_read_packet(uint8_t** audiobuf, int* audiobufsize, int* packetlength,
|
|
asf_waveformatex_t* wfx);
|
|
|
|
int asf_get_timestamp(int *duration);
|
|
|
|
int asf_seek(int ms, asf_waveformatex_t* wfx);
|
|
|
|
|
|
#endif /* _ASF_H */
|