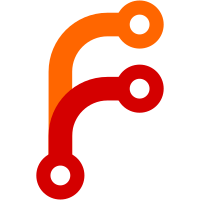
This library allows events to be subscribed / recieved within a lua script most events in rb are synchronous so flags are set and later checked by a secondary thread to make them (semi?) asynchronous. There are a few caveats to be aware of: FIRST, The main lua state is halted till the lua callback(s) are finished Yielding will not return control to your script from within a callback Also, subsequent callbacks may be delayed by the code in your lua callback SECOND, You must store the value returned from the event_register function you might get away with it for a bit but gc will destroy your callback eventually if you do not store the event THIRD, You only get one cb per event type ["action", "button", "custom", "playback", "timer"] (Re-registration of an event overwrites the previous one) Usage: possible events =["action", "button", "custom", "playback", "timer"] local evX = rockev.register("event", cb_function, [timeout / flags]) cb_function([id] [, data]) ... end rockev.suspend(["event"/nil][true/false]) passing nil affects all events stops event from executing, any but the last event before re-enabling will be lost, passing false, unregistering or re-registering an event will clear the suspend rockev.trigger("event", [true/false], [id]) sets an event to triggered, NOTE!, CUSTOM_EVENT must be unset manually id is only passed to callback by custom and playback events rockev.unregister(evX) Use unregister(evX) to remove an event Unregistering is not necessary before script end, it will be cleaned up on script exit Change-Id: Iea12a5cc0c0295b955dcc1cdf2eec835ca7e354d
55 lines
1.6 KiB
C
55 lines
1.6 KiB
C
/***************************************************************************
|
|
* __________ __ ___.
|
|
* Open \______ \ ____ ____ | | _\_ |__ _______ ___
|
|
* Source | _// _ \_/ ___\| |/ /| __ \ / _ \ \/ /
|
|
* Jukebox | | ( <_> ) \___| < | \_\ ( <_> > < <
|
|
* Firmware |____|_ /\____/ \___ >__|_ \|___ /\____/__/\_ \
|
|
* \/ \/ \/ \/ \/
|
|
* $Id$
|
|
*
|
|
* Copyright (C) 2008 Dan Everton (safetydan)
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This software is distributed on an "AS IS" basis, WITHOUT WARRANTY OF ANY
|
|
* KIND, either express or implied.
|
|
*
|
|
****************************************************************************/
|
|
|
|
#ifndef _ROCKLIB_H_
|
|
#define _ROCKLIB_H_
|
|
|
|
#define LUA_ROCKLIBNAME "rb"
|
|
|
|
#ifndef ERR_IDX_RANGE
|
|
#define ERR_IDX_RANGE "index out of range"
|
|
#endif
|
|
|
|
#ifndef ERR_DATA_OVF
|
|
#define ERR_DATA_OVF "data overflow"
|
|
#endif
|
|
|
|
#define RB_CONSTANT(x) {#x, x}
|
|
#define RB_STRING_CONSTANT(x) {#x, x}
|
|
|
|
struct lua_int_reg {
|
|
char const* name;
|
|
const int value;
|
|
};
|
|
|
|
struct lua_str_reg {
|
|
char const* name;
|
|
char const* value;
|
|
};
|
|
|
|
LUALIB_API int (luaopen_rock) (lua_State *L) __attribute__((aligned(0x8)));
|
|
/* in rockaux.c */
|
|
int get_current_path(lua_State *L, int level);
|
|
int filetol(int fd, long *num);
|
|
int get_plugin_action(int timeout, bool with_remote);
|
|
|
|
#endif /* _ROCKLIB_H_ */
|
|
|