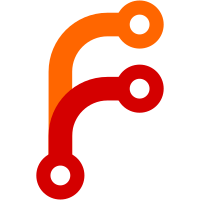
git-svn-id: svn://svn.rockbox.org/rockbox/trunk@13239 a1c6a512-1295-4272-9138-f99709370657
205 lines
5.1 KiB
C
205 lines
5.1 KiB
C
/***************************************************************************
|
|
* __________ __ ___.
|
|
* Open \______ \ ____ ____ | | _\_ |__ _______ ___
|
|
* Source | _// _ \_/ ___\| |/ /| __ \ / _ \ \/ /
|
|
* Jukebox | | ( <_> ) \___| < | \_\ ( <_> > < <
|
|
* Firmware |____|_ /\____/ \___ >__|_ \|___ /\____/__/\_ \
|
|
* \/ \/ \/ \/ \/
|
|
* $Id$
|
|
*
|
|
* Copyright (C) 2002 by Alan Korr
|
|
*
|
|
* All files in this archive are subject to the GNU General Public License.
|
|
* See the file COPYING in the source tree root for full license agreement.
|
|
*
|
|
* This software is distributed on an "AS IS" basis, WITHOUT WARRANTY OF ANY
|
|
* KIND, either express or implied.
|
|
*
|
|
****************************************************************************/
|
|
#include <stdio.h>
|
|
#include "config.h"
|
|
#include <stdbool.h>
|
|
#include "lcd.h"
|
|
#include "font.h"
|
|
#include "system.h"
|
|
#include "kernel.h"
|
|
#include "thread.h"
|
|
#include "timer.h"
|
|
#include "inttypes.h"
|
|
#include "string.h"
|
|
|
|
#ifndef SIMULATOR
|
|
long cpu_frequency NOCACHEBSS_ATTR = CPU_FREQ;
|
|
#endif
|
|
|
|
#ifdef HAVE_ADJUSTABLE_CPU_FREQ
|
|
static int boost_counter NOCACHEBSS_ATTR = 0;
|
|
static bool cpu_idle NOCACHEBSS_ATTR = false;
|
|
|
|
#if NUM_CORES > 1
|
|
struct mutex boostctrl_mtx NOCACHEBSS_ATTR;
|
|
#endif
|
|
|
|
int get_cpu_boost_counter(void)
|
|
{
|
|
return boost_counter;
|
|
}
|
|
#ifdef CPU_BOOST_LOGGING
|
|
#define MAX_BOOST_LOG 64
|
|
static char cpu_boost_calls[MAX_BOOST_LOG][MAX_PATH];
|
|
static int cpu_boost_first = 0;
|
|
static int cpu_boost_calls_count = 0;
|
|
static int cpu_boost_track_message = 0;
|
|
int cpu_boost_log_getcount(void)
|
|
{
|
|
return cpu_boost_calls_count;
|
|
}
|
|
char * cpu_boost_log_getlog_first(void)
|
|
{
|
|
if (cpu_boost_calls_count)
|
|
{
|
|
cpu_boost_track_message = 1;
|
|
return cpu_boost_calls[cpu_boost_first];
|
|
}
|
|
else return NULL;
|
|
}
|
|
char * cpu_boost_log_getlog_next(void)
|
|
{
|
|
int message = (cpu_boost_track_message+cpu_boost_first)%MAX_BOOST_LOG;
|
|
if (cpu_boost_track_message < cpu_boost_calls_count)
|
|
{
|
|
cpu_boost_track_message++;
|
|
return cpu_boost_calls[message];
|
|
}
|
|
else return NULL;
|
|
}
|
|
void cpu_boost_(bool on_off, char* location, int line)
|
|
{
|
|
if (cpu_boost_calls_count == MAX_BOOST_LOG)
|
|
{
|
|
cpu_boost_first = (cpu_boost_first+1)%MAX_BOOST_LOG;
|
|
cpu_boost_calls_count--;
|
|
if (cpu_boost_calls_count < 0)
|
|
cpu_boost_calls_count = 0;
|
|
}
|
|
if (cpu_boost_calls_count < MAX_BOOST_LOG)
|
|
{
|
|
int message = (cpu_boost_first+cpu_boost_calls_count)%MAX_BOOST_LOG;
|
|
snprintf(cpu_boost_calls[message], MAX_PATH,
|
|
"%c %s:%d",on_off==true?'B':'U',location,line);
|
|
cpu_boost_calls_count++;
|
|
}
|
|
#else
|
|
void cpu_boost(bool on_off)
|
|
{
|
|
#endif
|
|
if(on_off)
|
|
{
|
|
/* Boost the frequency if not already boosted */
|
|
if(boost_counter++ == 0)
|
|
set_cpu_frequency(CPUFREQ_MAX);
|
|
}
|
|
else
|
|
{
|
|
/* Lower the frequency if the counter reaches 0 */
|
|
if(--boost_counter == 0)
|
|
{
|
|
if(cpu_idle)
|
|
set_cpu_frequency(CPUFREQ_DEFAULT);
|
|
else
|
|
set_cpu_frequency(CPUFREQ_NORMAL);
|
|
}
|
|
|
|
/* Safety measure */
|
|
if(boost_counter < 0)
|
|
boost_counter = 0;
|
|
}
|
|
}
|
|
|
|
void cpu_idle_mode(bool on_off)
|
|
{
|
|
cpu_idle = on_off;
|
|
|
|
/* We need to adjust the frequency immediately if the CPU
|
|
isn't boosted */
|
|
if(boost_counter == 0)
|
|
{
|
|
if(cpu_idle)
|
|
set_cpu_frequency(CPUFREQ_DEFAULT);
|
|
else
|
|
set_cpu_frequency(CPUFREQ_NORMAL);
|
|
}
|
|
}
|
|
#endif /* HAVE_ADJUSTABLE_CPU_FREQ */
|
|
|
|
|
|
#ifdef HAVE_FLASHED_ROCKBOX
|
|
static bool detect_flash_header(uint8_t *addr)
|
|
{
|
|
#ifndef BOOTLOADER
|
|
int oldmode = system_memory_guard(MEMGUARD_NONE);
|
|
#endif
|
|
struct flash_header hdr;
|
|
memcpy(&hdr, addr, sizeof(struct flash_header));
|
|
#ifndef BOOTLOADER
|
|
system_memory_guard(oldmode);
|
|
#endif
|
|
return hdr.magic == FLASH_MAGIC;
|
|
}
|
|
#endif
|
|
|
|
bool detect_flashed_romimage(void)
|
|
{
|
|
#ifdef HAVE_FLASHED_ROCKBOX
|
|
return detect_flash_header((uint8_t *)FLASH_ROMIMAGE_ENTRY);
|
|
#else
|
|
return false;
|
|
#endif /* HAVE_FLASHED_ROCKBOX */
|
|
}
|
|
|
|
bool detect_flashed_ramimage(void)
|
|
{
|
|
#ifdef HAVE_FLASHED_ROCKBOX
|
|
return detect_flash_header((uint8_t *)FLASH_RAMIMAGE_ENTRY);
|
|
#else
|
|
return false;
|
|
#endif /* HAVE_FLASHED_ROCKBOX */
|
|
}
|
|
|
|
bool detect_original_firmware(void)
|
|
{
|
|
return !(detect_flashed_ramimage() || detect_flashed_romimage());
|
|
}
|
|
|
|
#if defined(CPU_ARM)
|
|
|
|
static const char* const uiename[] = {
|
|
"Undefined instruction", "Prefetch abort", "Data abort"
|
|
};
|
|
|
|
/* Unexpected Interrupt or Exception handler. Currently only deals with
|
|
exceptions, but will deal with interrupts later.
|
|
*/
|
|
void UIE(unsigned int pc, unsigned int num)
|
|
{
|
|
char str[32];
|
|
|
|
lcd_clear_display();
|
|
#ifdef HAVE_LCD_BITMAP
|
|
lcd_setfont(FONT_SYSFIXED);
|
|
#endif
|
|
lcd_puts(0, 0, uiename[num]);
|
|
snprintf(str, sizeof(str), "at %08x", pc);
|
|
lcd_puts(0, 1, str);
|
|
lcd_update();
|
|
|
|
while (1)
|
|
{
|
|
/* TODO: perhaps add button handling in here when we get a polling
|
|
driver some day.
|
|
*/
|
|
}
|
|
}
|
|
|
|
#endif /* CPU_ARM */
|
|
|