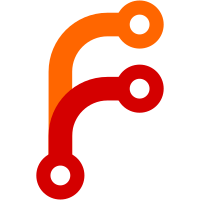
git-svn-id: svn://svn.rockbox.org/rockbox/trunk@13906 a1c6a512-1295-4272-9138-f99709370657
288 lines
9 KiB
C++
288 lines
9 KiB
C++
/***************************************************************************
|
|
* __________ __ ___.
|
|
* Open \______ \ ____ ____ | | _\_ |__ _______ ___
|
|
* Source | _// _ \_/ ___\| |/ /| __ \ / _ \ \/ /
|
|
* Jukebox | | ( <_> ) \___| < | \_\ ( <_> > < <
|
|
* Firmware |____|_ /\____/ \___ >__|_ \|___ /\____/__/\_ \
|
|
* \/ \/ \/ \/ \/
|
|
* Module: rbutil
|
|
* File: rbutilApp.cpp
|
|
*
|
|
* Copyright (C) 2005 Christi Alice Scarborough
|
|
*
|
|
* All files in this archive are subject to the GNU General Public License.
|
|
* See the file COPYING in the source tree root for full license agreement.
|
|
*
|
|
* This software is distributed on an "AS IS" basis, WITHOUT WARRANTY OF ANY
|
|
* KIND, either express or implied.
|
|
*
|
|
****************************************************************************/
|
|
|
|
#include "rbutilApp.h"
|
|
#include "bootloaders.h"
|
|
|
|
GlobalVars* gv = new GlobalVars();
|
|
|
|
IMPLEMENT_APP(rbutilFrmApp)
|
|
|
|
bool rbutilFrmApp::OnInit()
|
|
{
|
|
wxString buf = wxT("");
|
|
|
|
wxLogVerbose(wxT("=== begin rbutilFrmApp::Oninit()"));
|
|
|
|
|
|
gv->stdpaths = new wxStandardPaths();
|
|
|
|
// Get application directory
|
|
// DANGER! GetDataDir() doesn't portably return the application directory
|
|
// We want to use the form below instead, but not until wxWidgets 2.8 is
|
|
// released. *Datadir gives the wrong dir for this on Linux/Mac even on Wx2.8 *
|
|
gv->AppDir = gv->stdpaths->GetExecutablePath().BeforeLast(PATH_SEP_CHR);
|
|
// buf = gv->stdpaths->GetDataDir(); buf.Append(PATH_SEP);
|
|
// gv->AppDir = buf.BeforeLast(PATH_SEP_CHR).c_str();
|
|
|
|
buf = gv->stdpaths->GetUserDataDir();
|
|
if (! wxDirExists(buf) )
|
|
{
|
|
wxLogNull lognull;
|
|
if (! wxMkdir(buf, 0777))
|
|
{
|
|
wxLogFatalError(wxT("Can't create data directory %s"),
|
|
buf.c_str());
|
|
}
|
|
}
|
|
|
|
buf += PATH_SEP wxT("rbutil.log");
|
|
gv->logfile = new wxFFile(buf, wxT("w"));
|
|
if (! gv->logfile->IsOpened() )
|
|
wxLogFatalError(wxT("Unable to open log file"));
|
|
|
|
gv->loggui = new wxLogGui();
|
|
gv->loggui->SetActiveTarget(gv->loggui);
|
|
gv->loggui->SetLogLevel(wxLOG_Message);
|
|
gv->logchain = new wxLogChain(
|
|
gv->logstderr = new wxLogStderr(gv->logfile->fp() ) );
|
|
|
|
buf = buf.Left(buf.Len() - 10);
|
|
buf.Append(wxT("download"));
|
|
if (! wxDirExists(buf) ) wxMkdir(buf, 0777);
|
|
|
|
wxFileSystem::AddHandler(new wxInternetFSHandler);
|
|
wxFileSystem::AddHandler(new wxZipFSHandler);
|
|
|
|
if (!ReadGlobalConfig(NULL))
|
|
{
|
|
ERR_DIALOG(gv->ErrStr->GetData(), wxT("Rockbox Utility"));
|
|
return FALSE;
|
|
}
|
|
ReadUserConfig();
|
|
|
|
wxInitAllImageHandlers(); //init Image handlers
|
|
initIpodpatcher(); // reserve mem for ipodpatcher
|
|
initSansaPatcher(); // reserve mem for sansapatcher
|
|
|
|
rbutilFrm *myFrame = new rbutilFrm(NULL);
|
|
SetTopWindow(myFrame);
|
|
|
|
myFrame->Show(TRUE);
|
|
|
|
wxLogVerbose(wxT("=== end rbUtilFrmApp::OnInit()"));
|
|
return TRUE;
|
|
}
|
|
|
|
int rbutilFrmApp::OnExit()
|
|
{
|
|
wxLogVerbose(wxT("=== begin rbUtilFrmApp::OnExit()"));
|
|
|
|
WriteUserConfig();
|
|
|
|
gv->logfile->Close();
|
|
/* Enabling this code causes the program to crash. I
|
|
* have no idea why. (possibly because deleting non existing objects ? :-) )
|
|
wxLog::DontCreateOnDemand();
|
|
// Free a bunch of structures.
|
|
delete gv->GlobalConfig;
|
|
delete gv->ErrStr;
|
|
delete gv->stdpaths;
|
|
delete gv->platform;
|
|
|
|
delete gv->logstderr;
|
|
delete gv->logchain;
|
|
delete gv->logfile;
|
|
delete gv->loggui;
|
|
*/
|
|
wxLogVerbose(wxT("=== end rbUtilFrmApp::OnExit()"));
|
|
return 0;
|
|
}
|
|
|
|
bool rbutilFrmApp::ReadGlobalConfig(rbutilFrm* myFrame)
|
|
{
|
|
wxString buf, tmpstr, stack;
|
|
wxLogVerbose(wxT("=== begin rbutilFrmApp::ReadGlobalConfig(%p)"),
|
|
(void*) myFrame);
|
|
|
|
// Cross-platform compatibility: look for rbutil.ini in then in the app dir
|
|
// then in the user config dir (linux ~/) and
|
|
// then config dir (linux /etc/ )
|
|
|
|
buf = gv->AppDir + wxT("" PATH_SEP "rbutil.ini");
|
|
if (! wxFileExists(buf) )
|
|
{
|
|
buf = gv->stdpaths->GetUserConfigDir()
|
|
+ wxT("" PATH_SEP ".rbutil" PATH_SEP "rbutil.ini");
|
|
if (! wxFileExists(buf) )
|
|
{
|
|
buf = gv->stdpaths->GetConfigDir()
|
|
+ wxT("" PATH_SEP "rbutil.ini");
|
|
}
|
|
}
|
|
|
|
if (! wxFileExists(buf) )
|
|
{
|
|
gv->ErrStr = new wxString(wxT("Configuration file doesnt exist!"));
|
|
return false;
|
|
}
|
|
|
|
|
|
wxFileInputStream* cfgis = new wxFileInputStream(buf);
|
|
|
|
if (!cfgis->CanRead()) {
|
|
gv->ErrStr = new wxString(wxT("Unable to open configuration file"));
|
|
return false;
|
|
}
|
|
|
|
gv->GlobalConfig = new wxFileConfig(*cfgis);
|
|
gv->GlobalConfigFile = buf;
|
|
|
|
unsigned int i = 0;
|
|
|
|
stack = gv->GlobalConfig->GetPath();
|
|
gv->GlobalConfig->SetPath(wxT("/platforms"));
|
|
while(gv->GlobalConfig->Read(buf.Format(wxT("platform%d"), i + 1),
|
|
&tmpstr)) {
|
|
wxString cur = tmpstr;
|
|
//gv->plat_id.Add(tmpstr);
|
|
gv->GlobalConfig->Read(buf.Format(wxT("/%s/name"),
|
|
cur.c_str()), &tmpstr);
|
|
gv->plat_name.Add(tmpstr);
|
|
gv->GlobalConfig->Read(buf.Format(wxT("/%s/platform"),
|
|
cur.c_str()), &tmpstr);
|
|
gv->plat_id.Add(tmpstr);
|
|
gv->GlobalConfig->Read(buf.Format(wxT("/%s/released"),
|
|
cur.c_str()), &tmpstr);
|
|
gv->plat_released.Add( (tmpstr == wxT("yes")) ? true : false ) ;
|
|
gv->GlobalConfig->Read(buf.Format(wxT("/%s/needsbootloader"),
|
|
cur.c_str()), &tmpstr);
|
|
gv->plat_needsbootloader.Add( (tmpstr == wxT("yes")) ? true : false ) ;
|
|
gv->GlobalConfig->Read(buf.Format(wxT("/%s/bootloadermethod"),
|
|
cur.c_str()), &tmpstr);
|
|
gv->plat_bootloadermethod.Add(tmpstr);
|
|
gv->GlobalConfig->Read(buf.Format(wxT("/%s/bootloadername"),
|
|
cur.c_str()), &tmpstr);
|
|
gv->plat_bootloadername.Add(tmpstr);
|
|
gv->GlobalConfig->Read(buf.Format(wxT("/%s/resolution"),
|
|
cur.c_str()), &tmpstr);
|
|
gv->plat_resolution.Add(tmpstr);
|
|
gv->GlobalConfig->Read(buf.Format(wxT("/%s/manualname"),
|
|
cur.c_str()), &tmpstr);
|
|
gv->plat_manualname.Add(tmpstr);
|
|
|
|
i++;
|
|
}
|
|
|
|
gv->GlobalConfig->SetPath(wxT("/general"));
|
|
gv->GlobalConfig->Read(wxT("default_platform"), &tmpstr, wxT("cthulhu"));
|
|
|
|
|
|
gv->GlobalConfig->Read(wxT("last_release"), &tmpstr);
|
|
gv->last_release = tmpstr;
|
|
|
|
gv->GlobalConfig->Read(wxT("download_url"), &tmpstr);
|
|
gv->download_url = tmpstr;
|
|
|
|
gv->GlobalConfig->Read(wxT("daily_url"), &tmpstr);
|
|
gv->daily_url = tmpstr;
|
|
|
|
gv->GlobalConfig->Read(wxT("bleeding_url"), &tmpstr);
|
|
gv->bleeding_url = tmpstr;
|
|
|
|
gv->GlobalConfig->Read(wxT("server_conf_url"), &tmpstr);
|
|
gv->server_conf_url = tmpstr;
|
|
|
|
gv->GlobalConfig->Read(wxT("font_url"), &tmpstr);
|
|
gv->font_url = tmpstr;
|
|
|
|
gv->GlobalConfig->Read(wxT("prog_name"), &tmpstr);
|
|
gv->prog_name = tmpstr;
|
|
|
|
gv->GlobalConfig->Read(wxT("bootloader_url"), &tmpstr);
|
|
gv->bootloader_url = tmpstr;
|
|
|
|
gv->GlobalConfig->Read(wxT("themes_url"), &tmpstr);
|
|
gv->themes_url = tmpstr;
|
|
|
|
gv->GlobalConfig->Read(wxT("manual_url"), &tmpstr);
|
|
gv->manual_url = tmpstr;
|
|
|
|
gv->GlobalConfig->Read(wxT("doom_url"), &tmpstr);
|
|
gv->doom_url = tmpstr;
|
|
|
|
#ifdef __WXMSW__
|
|
gv->curdestdir = wxT("D:\\");
|
|
#else
|
|
gv->curdestdir = wxT("/mnt");
|
|
#endif
|
|
gv->GlobalConfig->SetPath(stack);
|
|
|
|
wxLogVerbose(wxT("=== end rbutilFrmApp::ReadGlobalConfig()"));
|
|
return true;
|
|
}
|
|
|
|
void rbutilFrmApp::ReadUserConfig()
|
|
{
|
|
wxString buf, str, stack;
|
|
|
|
buf = gv->AppDir + wxT("" PATH_SEP "RockboxUtility.cfg");
|
|
|
|
if (wxFileExists(buf) )
|
|
{
|
|
gv->portable = true;
|
|
}
|
|
else
|
|
{
|
|
gv->portable = false;
|
|
buf = gv->stdpaths->GetUserDataDir()
|
|
+ wxT("" PATH_SEP "RockboxUtility.cfg");
|
|
}
|
|
|
|
gv->UserConfig = new wxFileConfig(wxEmptyString, wxEmptyString, buf);
|
|
gv->UserConfigFile = buf;
|
|
gv->UserConfig->Set(gv->UserConfig); // Store wxWidgets internal settings
|
|
stack = gv->UserConfig->GetPath();
|
|
|
|
gv->UserConfig->SetPath(wxT("/defaults"));
|
|
if (gv->UserConfig->Read(wxT("curdestdir"), &str) ) gv->curdestdir = str;
|
|
if (gv->UserConfig->Read(wxT("curplatform"), &str) ) gv->curplat = str;
|
|
if (gv->UserConfig->Read(wxT("curfirmware"), &str) ) gv->curfirmware = str;
|
|
if (gv->UserConfig->Read(wxT("proxy_url"), &str) ) gv->proxy_url = str;
|
|
|
|
if (gv->UserConfig->Read(wxT("pathToTts"), &str) ) gv->pathToTts = str;
|
|
if (gv->UserConfig->Read(wxT("pathToEnc"), &str) ) gv->pathToEnc = str;
|
|
gv->UserConfig->SetPath(stack);
|
|
}
|
|
|
|
void rbutilFrmApp::WriteUserConfig()
|
|
{
|
|
gv->UserConfig->SetPath(wxT("/defaults"));
|
|
gv->UserConfig->Write(wxT("curdestdir"), gv->curdestdir);
|
|
gv->UserConfig->Write(wxT("curplatform"), gv->curplat);
|
|
gv->UserConfig->Write(wxT("curfirmware"), gv->curfirmware);
|
|
gv->UserConfig->Write(wxT("proxy_url"), gv->proxy_url);
|
|
gv->UserConfig->Write(wxT("pathToTts"), gv->pathToTts);
|
|
gv->UserConfig->Write(wxT("pathToEnc"), gv->pathToEnc);
|
|
|
|
delete gv->UserConfig;
|
|
|
|
}
|
|
|