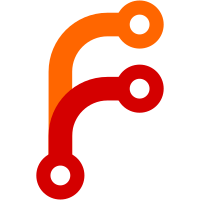
later. We still need to hunt down snippets used that are not. 1324 modified files... http://www.rockbox.org/mail/archive/rockbox-dev-archive-2008-06/0060.shtml git-svn-id: svn://svn.rockbox.org/rockbox/trunk@17847 a1c6a512-1295-4272-9138-f99709370657
121 lines
2.6 KiB
C
121 lines
2.6 KiB
C
/***************************************************************************
|
|
* __________ __ ___.
|
|
* Open \______ \ ____ ____ | | _\_ |__ _______ ___
|
|
* Source | _// _ \_/ ___\| |/ /| __ \ / _ \ \/ /
|
|
* Jukebox | | ( <_> ) \___| < | \_\ ( <_> > < <
|
|
* Firmware |____|_ /\____/ \___ >__|_ \|___ /\____/__/\_ \
|
|
* \/ \/ \/ \/ \/
|
|
* $Id$
|
|
*
|
|
* Copyright (C) 2002 by Linus Nielsen Feltzing
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This software is distributed on an "AS IS" basis, WITHOUT WARRANTY OF ANY
|
|
* KIND, either express or implied.
|
|
*
|
|
****************************************************************************/
|
|
#include "lcd.h"
|
|
#include "sh7034.h"
|
|
#include "kernel.h"
|
|
#include "thread.h"
|
|
#include "debug.h"
|
|
#include "system.h"
|
|
#include "fmradio.h"
|
|
|
|
#if CONFIG_TUNER
|
|
|
|
/* Signals:
|
|
DI (Data In) - PB0 (doubles as data pin for the LCD)
|
|
CL (Clock) - PB1 (doubles as clock for the LCD)
|
|
CE (Chip Enable) - PB3 (also chip select for the LCD, but active low)
|
|
DO (Data Out) - PB4
|
|
*/
|
|
|
|
/* cute little functions */
|
|
#define CE_LO and_b(~0x08, PBDRL_ADDR)
|
|
#define CE_HI or_b(0x08, PBDRL_ADDR)
|
|
#define CL_LO and_b(~0x02, PBDRL_ADDR)
|
|
#define CL_HI or_b(0x02, PBDRL_ADDR)
|
|
#define DO (PBDR & 0x10)
|
|
#define DI_LO and_b(~0x01, PBDRL_ADDR)
|
|
#define DI_HI or_b(0x01, PBDRL_ADDR)
|
|
|
|
#define START or_b((0x08 | 0x02), PBDRL_ADDR)
|
|
|
|
/* delay loop */
|
|
#define DELAY do { int _x; for(_x=0;_x<10;_x++);} while (0)
|
|
|
|
|
|
int fmradio_read(int addr)
|
|
{
|
|
int i;
|
|
int data = 0;
|
|
|
|
START;
|
|
|
|
/* First address bit */
|
|
CL_LO;
|
|
if(addr & 2)
|
|
DI_HI;
|
|
else
|
|
DI_LO;
|
|
DELAY;
|
|
CL_HI;
|
|
DELAY;
|
|
|
|
/* Second address bit */
|
|
CL_LO;
|
|
if(addr & 1)
|
|
DI_HI;
|
|
else
|
|
DI_LO;
|
|
DELAY;
|
|
CL_HI;
|
|
DELAY;
|
|
|
|
for(i = 0; i < 21;i++)
|
|
{
|
|
CL_LO;
|
|
DELAY;
|
|
data <<= 1;
|
|
data |= (DO)?1:0;
|
|
CL_HI;
|
|
DELAY;
|
|
}
|
|
|
|
CE_LO;
|
|
|
|
return data;
|
|
}
|
|
|
|
void fmradio_set(int addr, int data)
|
|
{
|
|
int i;
|
|
|
|
/* Include the address in the data */
|
|
data |= addr << 21;
|
|
|
|
START;
|
|
|
|
for(i = 0; i < 23;i++)
|
|
{
|
|
CL_LO;
|
|
DELAY;
|
|
if(data & (1 << 22))
|
|
DI_HI;
|
|
else
|
|
DI_LO;
|
|
|
|
data <<= 1;
|
|
CL_HI;
|
|
DELAY;
|
|
}
|
|
|
|
CE_LO;
|
|
}
|
|
|
|
#endif
|