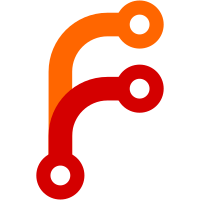
1) Introduces apps/features.txt that controls which strings are included for each target based on defines. 2) .lng and .voice files are now target specific and the format versions of both these file types have been bumped, which means that new voice files are needed. 3) Use the 'features' mechanism to exclude strings for targets that didn't use them. 4) Delete unused and deprecated and duplicated strings, sort strings in english.lang Some string IDs were changed so translations will be slightly worse than before. git-svn-id: svn://svn.rockbox.org/rockbox/trunk@14198 a1c6a512-1295-4272-9138-f99709370657
91 lines
2.9 KiB
C
91 lines
2.9 KiB
C
/***************************************************************************
|
|
* __________ __ ___.
|
|
* Open \______ \ ____ ____ | | _\_ |__ _______ ___
|
|
* Source | _// _ \_/ ___\| |/ /| __ \ / _ \ \/ /
|
|
* Jukebox | | ( <_> ) \___| < | \_\ ( <_> > < <
|
|
* Firmware |____|_ /\____/ \___ >__|_ \|___ /\____/__/\_ \
|
|
* \/ \/ \/ \/ \/
|
|
* $Id$
|
|
*
|
|
* Copyright (C) 2002 Daniel Stenberg
|
|
*
|
|
* All files in this archive are subject to the GNU General Public License.
|
|
* See the file COPYING in the source tree root for full license agreement.
|
|
*
|
|
* This software is distributed on an "AS IS" basis, WITHOUT WARRANTY OF ANY
|
|
* KIND, either express or implied.
|
|
*
|
|
****************************************************************************/
|
|
|
|
#include <file.h>
|
|
#if defined(SIMULATOR) && defined(__MINGW32__)
|
|
extern int printf(const char *format, ...);
|
|
#endif
|
|
|
|
#include "language.h"
|
|
#include "lang.h"
|
|
#include "debug.h"
|
|
#include "string.h"
|
|
|
|
static unsigned char language_buffer[MAX_LANGUAGE_SIZE];
|
|
|
|
void lang_init(void)
|
|
{
|
|
int i;
|
|
unsigned char *ptr = (unsigned char *) language_builtin;
|
|
|
|
for (i = 0; i < LANG_LAST_INDEX_IN_ARRAY; i++) {
|
|
language_strings[i] = ptr;
|
|
ptr += strlen((char *)ptr) + 1; /* advance pointer to next string */
|
|
}
|
|
}
|
|
|
|
int lang_load(const char *filename)
|
|
{
|
|
int fsize;
|
|
int fd = open(filename, O_RDONLY);
|
|
int retcode=0;
|
|
unsigned char lang_header[3];
|
|
if(fd == -1)
|
|
return 1;
|
|
fsize = filesize(fd) - 2;
|
|
if(fsize <= MAX_LANGUAGE_SIZE) {
|
|
read(fd, lang_header, 3);
|
|
if((lang_header[0] == LANGUAGE_COOKIE) &&
|
|
(lang_header[1] == LANGUAGE_VERSION) &&
|
|
(lang_header[2] == TARGET_ID)) {
|
|
read(fd, language_buffer, MAX_LANGUAGE_SIZE);
|
|
unsigned char *ptr = language_buffer;
|
|
int id;
|
|
lang_init(); /* initialize with builtin */
|
|
|
|
while(fsize>3) {
|
|
id = (ptr[0]<<8) | ptr[1]; /* get two-byte id */
|
|
ptr+=2; /* pass the id */
|
|
if(id < LANG_LAST_INDEX_IN_ARRAY) {
|
|
#if 0
|
|
printf("%2x New: %30s ", id, ptr);
|
|
printf("Replaces: %s\n", language_strings[id]);
|
|
#endif
|
|
language_strings[id] = ptr; /* point to this string */
|
|
}
|
|
while(*ptr) { /* pass the string */
|
|
fsize--;
|
|
ptr++;
|
|
}
|
|
fsize-=3; /* the id and the terminating zero */
|
|
ptr++; /* pass the terminating zero-byte */
|
|
}
|
|
}
|
|
else {
|
|
DEBUGF("Illegal language file\n");
|
|
retcode = 2;
|
|
}
|
|
}
|
|
else {
|
|
DEBUGF("Language %s too large: %d\n", filename, fsize);
|
|
retcode = 3;
|
|
}
|
|
close(fd);
|
|
return retcode;
|
|
}
|