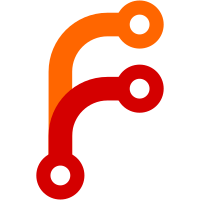
I'm currently running up against the limitations of the lcd_draw functions I want these functions to be able to be used on any size buffer not just buffers with a stride matching the underlying device [DONE] allow the framebuffer to be decoupled from the device framebuffer [DONE need examples] allow for some simple blit like transformations [DONE] remove the device framebuffer from the plugin api [DONE}ditto remote framebuffer [DONE] remove _viewport_get_framebuffer you can call struct *vp = lcd_set_viewport(NULL) and vp->buffer->fb_ptr while remote lcds may compile (and work in the sim) its not been tested on targets [FIXED] backdrops need work to be screen agnostic [FIXED] screen statusbar is not being combined into the main viewport correctly yet [FIXED] screen elements are displayed incorrectly after switch to void* [FIXED] core didn't restore proper viewport on splash etc. [NEEDS TESTING] remote lcd garbled data [FIXED] osd lib garbled screen on bmp_part [FIXED] grey_set_vp needs to return old viewport like lcd_set_viewport [FIXED] Viewport update now handles viewports with differing buffers/strides by copying to the main buffer [FIXED] splash on top of WPS leaves old framebuffer data (doesn't redraw) [UPDATE] refined this a bit more to have clear_viewport set the clean bit and have skin_render do its own screen clear scrolling viewports no longer trigger wps refresh also fixed a bug where guisyncyesno was displaying and then disappearing [ADDED!] New LCD macros that allow you to create properly size frame buffers in you desired size without wasting bytes (LCD_ and LCD_REMOTE_) LCD_STRIDE(w, h) same as STRIDE_MAIN LCD_FBSTRIDE(w, h) returns target specific stride for a buffer W x H LCD_NBELEMS(w, h) returns the number of fb_data sized elemenst needed for a buffer W x H LCD_NATIVE_STRIDE(s) conversion between rockbox native vertical and lcd native stride (2bitH) test_viewports.c has an example of usage [FIXED!!] 2bit targets don't respect non-native strides [FIXED] Few define snags Change-Id: I0d04c3834e464eca84a5a715743a297a0cefd0af
102 lines
3 KiB
C
102 lines
3 KiB
C
#include "zxvid_com.h"
|
|
|
|
/* screen routines for color targets */
|
|
|
|
#define N0 0x00
|
|
#define N1 0xC0
|
|
#define B0 0x00
|
|
#define B1 0xFF
|
|
|
|
#define IN0 (0xFF-N0)
|
|
#define IN1 (0xFF-N1)
|
|
#define IB0 (0xFF-B0)
|
|
#define IB1 (0xFF-B1)
|
|
|
|
static const unsigned _16bpp_colors[32] = {
|
|
/* normal */
|
|
LCD_RGBPACK(N0, N0, N0), LCD_RGBPACK(N0, N0, N1),
|
|
LCD_RGBPACK(N1, N0, N0), LCD_RGBPACK(N1, N0, N1),
|
|
LCD_RGBPACK(N0, N1, N0), LCD_RGBPACK(N0, N1, N1),
|
|
LCD_RGBPACK(N1, N1, N0), LCD_RGBPACK(N1, N1, N1),
|
|
LCD_RGBPACK(B0, B0, B0), LCD_RGBPACK(B0, B0, B1),
|
|
LCD_RGBPACK(B1, B0, B0), LCD_RGBPACK(B1, B0, B1),
|
|
LCD_RGBPACK(B0, B1, B0), LCD_RGBPACK(B0, B1, B1),
|
|
LCD_RGBPACK(B1, B1, B0), LCD_RGBPACK(B1, B1, B1),
|
|
/* inverted */
|
|
LCD_RGBPACK(IN0, IN0, IN0), LCD_RGBPACK(IN0, IN0, IN1),
|
|
LCD_RGBPACK(IN1, IN0, IN0), LCD_RGBPACK(IN1, IN0, IN1),
|
|
LCD_RGBPACK(IN0, IN1, IN0), LCD_RGBPACK(IN0, IN1, IN1),
|
|
LCD_RGBPACK(IN1, IN1, IN0), LCD_RGBPACK(IN1, IN1, IN1),
|
|
LCD_RGBPACK(IB0, IB0, IB0), LCD_RGBPACK(IB0, IB0, IB1),
|
|
LCD_RGBPACK(IB1, IB0, IB0), LCD_RGBPACK(IB1, IB0, IB1),
|
|
LCD_RGBPACK(IB0, IB1, IB0), LCD_RGBPACK(IB0, IB1, IB1),
|
|
LCD_RGBPACK(IB1, IB1, IB0), LCD_RGBPACK(IB1, IB1, IB1),
|
|
};
|
|
|
|
static fb_data *lcd_fb = NULL;
|
|
|
|
void init_spect_scr(void)
|
|
{
|
|
int i;
|
|
int offset = settings.invert_colors ? 16 : 0;
|
|
|
|
for(i = 0; i < 16; i++)
|
|
sp_colors[i] = i + offset;
|
|
|
|
sp_image = (char *) &image_array;
|
|
spscr_init_mask_color();
|
|
spscr_init_line_pointers(HEIGHT);
|
|
|
|
}
|
|
|
|
void update_screen(void)
|
|
{
|
|
if (!lcd_fb)
|
|
{
|
|
struct viewport *vp_main = rb->lcd_set_viewport(NULL);
|
|
lcd_fb = vp_main->buffer->fb_ptr;
|
|
}
|
|
fb_data *frameb;
|
|
|
|
int y=0;
|
|
|
|
#if LCD_HEIGHT >= ZX_HEIGHT && LCD_WIDTH >= ZX_WIDTH
|
|
/* 'set but not used'
|
|
byte *scrptr;
|
|
scrptr = (byte *) SPNM(image);
|
|
*/
|
|
frameb = lcd_fb;
|
|
for ( y = 0 ; y < HEIGHT*WIDTH; y++ ){
|
|
frameb[y] = FB_SCALARPACK(_16bpp_colors[(unsigned)sp_image[y]]);
|
|
}
|
|
|
|
#else
|
|
int x=0;
|
|
int srcx, srcy=0; /* x / y coordinates in source image */
|
|
unsigned char* image;
|
|
image = sp_image + ( (Y_OFF)*(WIDTH) ) + X_OFF;
|
|
frameb = lcd_fb;
|
|
for(y = 0; y < LCD_HEIGHT; y++)
|
|
{
|
|
srcx = 0; /* reset our x counter before each row... */
|
|
for(x = 0; x < LCD_WIDTH; x++)
|
|
{
|
|
*frameb = FB_SCALARPACK(_16bpp_colors[image[srcx>>16]]);
|
|
srcx += X_STEP; /* move through source image */
|
|
frameb++;
|
|
}
|
|
srcy += Y_STEP; /* move through the source image... */
|
|
image += (srcy>>16)*WIDTH; /* and possibly to the next row. */
|
|
srcy &= 0xffff; /* set up the y-coordinate between 0 and 1 */
|
|
}
|
|
|
|
#endif
|
|
if ( settings.showfps ) {
|
|
int percent=0;
|
|
int TPF = HZ/50;/* ticks per frame */
|
|
if ((*rb->current_tick-start_time) > TPF )
|
|
percent = 100*video_frames/((*rb->current_tick-start_time)/TPF);
|
|
rb->lcd_putsxyf(0,0,"%d %%",percent);
|
|
}
|
|
rb -> lcd_update();
|
|
}
|