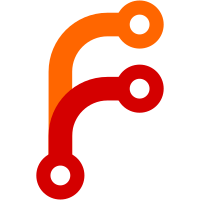
Part of this change is to align sdlapp builds to other application targets in that the sim_* wrappers are not used anymore (except for sim_read/write). Path mangling is now done in rbpaths.c as well. Change-Id: I9726da73b50a83d9e1a1840288de16ec01ea029d
107 lines
2.9 KiB
C
107 lines
2.9 KiB
C
/***************************************************************************
|
|
* __________ __ ___.
|
|
* Open \______ \ ____ ____ | | _\_ |__ _______ ___
|
|
* Source | _// _ \_/ ___\| |/ /| __ \ / _ \ \/ /
|
|
* Jukebox | | ( <_> ) \___| < | \_\ ( <_> > < <
|
|
* Firmware |____|_ /\____/ \___ >__|_ \|___ /\____/__/\_ \
|
|
* \/ \/ \/ \/ \/
|
|
* $Id: dir.h 13741 2007-06-30 02:08:27Z jethead71 $
|
|
*
|
|
* Copyright (C) 2002 by Björn Stenberg
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This software is distributed on an "AS IS" basis, WITHOUT WARRANTY OF ANY
|
|
* KIND, either express or implied.
|
|
*
|
|
****************************************************************************/
|
|
#ifndef _DIR_UNCACHED_H_
|
|
#define _DIR_UNCACHED_H_
|
|
|
|
#include "config.h"
|
|
|
|
struct dirinfo {
|
|
int attribute;
|
|
long size;
|
|
unsigned short wrtdate;
|
|
unsigned short wrttime;
|
|
};
|
|
|
|
#include <stdbool.h>
|
|
#include "file.h"
|
|
|
|
#if defined(SIMULATOR) || defined(__PCTOOL__)
|
|
# define dirent_uncached sim_dirent
|
|
# define DIR_UNCACHED SIM_DIR
|
|
# define opendir_uncached sim_opendir
|
|
# define readdir_uncached sim_readdir
|
|
# define closedir_uncached sim_closedir
|
|
# define mkdir_uncached sim_mkdir
|
|
# define rmdir_uncached sim_rmdir
|
|
#elif defined(APPLICATION)
|
|
# include "rbpaths.h"
|
|
# define DIRENT_DEFINED
|
|
# define DIR_DEFINED
|
|
# define dirent_uncached dirent
|
|
# define DIR_UNCACHED DIR
|
|
# define opendir_uncached app_opendir
|
|
# define readdir_uncached app_readdir
|
|
# define closedir_uncached app_closedir
|
|
# define mkdir_uncached app_mkdir
|
|
# define rmdir_uncached app_rmdir
|
|
#endif
|
|
|
|
|
|
#ifndef DIRENT_DEFINED
|
|
struct dirent_uncached {
|
|
unsigned char d_name[MAX_PATH];
|
|
struct dirinfo info;
|
|
long startcluster;
|
|
};
|
|
#endif
|
|
|
|
#include "fat.h"
|
|
|
|
#ifndef DIR_DEFINED
|
|
typedef struct {
|
|
#if (CONFIG_PLATFORM & PLATFORM_NATIVE)
|
|
struct fat_dir fatdir CACHEALIGN_ATTR;
|
|
bool busy;
|
|
long startcluster;
|
|
struct dirent_uncached theent;
|
|
#ifdef HAVE_MULTIVOLUME
|
|
int volumecounter; /* running counter for faked volume entries */
|
|
#endif
|
|
#else
|
|
/* simulator/application: */
|
|
void *dir; /* actually a DIR* dir */
|
|
char *name;
|
|
#endif
|
|
} DIR_UNCACHED CACHEALIGN_ATTR;
|
|
#endif
|
|
|
|
#ifdef HAVE_HOTSWAP
|
|
char *get_volume_name(int volume);
|
|
#endif
|
|
|
|
#ifdef HAVE_MULTIVOLUME
|
|
int strip_volume(const char*, char*);
|
|
#endif
|
|
|
|
#ifndef DIRFUNCTIONS_DEFINED
|
|
|
|
extern DIR_UNCACHED* opendir_uncached(const char* name);
|
|
extern int closedir_uncached(DIR_UNCACHED* dir);
|
|
extern int mkdir_uncached(const char* name);
|
|
extern int rmdir_uncached(const char* name);
|
|
|
|
extern struct dirent_uncached* readdir_uncached(DIR_UNCACHED* dir);
|
|
|
|
extern int release_dirs(int volume);
|
|
|
|
#endif /* DIRFUNCTIONS_DEFINED */
|
|
|
|
#endif
|