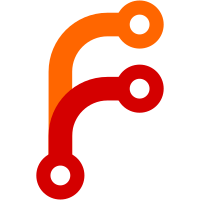
With the new lcd_putsxy_scroll_func() code can register custom scroll functions (put_line() makes use of that). In order for the custom scroller to be able to properly manage its userdata pointer (set via struct scrollinfo::userdata) the scroll engine must inform the scroller about start and stop of scrolling. To inform about start the lcd_scroll_* functions now return true when the line will scroll. To inform about stop the scroll engine calls into the scroller one last time, with the text set to NULL. put_line() can use this to release the userdata registered per scrolling line so that it can be recycled. This fixes that some scrolling lines became glitchy after some time because the userdata was recycled too early. Change-Id: Iff0a6ce2a4f9ae2bada1b8e62f4f5950224942a9
126 lines
4.2 KiB
C
126 lines
4.2 KiB
C
/***************************************************************************
|
|
* __________ __ ___.
|
|
* Open \______ \ ____ ____ | | _\_ |__ _______ ___
|
|
* Source | _// _ \_/ ___\| |/ /| __ \ / _ \ \/ /
|
|
* Jukebox | | ( <_> ) \___| < | \_\ ( <_> > < <
|
|
* Firmware |____|_ /\____/ \___ >__|_ \|___ /\____/__/\_ \
|
|
* \/ \/ \/ \/ \/
|
|
* $Id$
|
|
*
|
|
* Copyright (C) 2007 Michael Sevakis
|
|
*
|
|
* LCD scrolling driver and scheduler
|
|
*
|
|
* Much collected and combined from the various Rockbox LCD drivers.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This software is distributed on an "AS IS" basis, WITHOUT WARRANTY OF ANY
|
|
* KIND, either express or implied.
|
|
*
|
|
****************************************************************************/
|
|
#ifndef __SCROLL_ENGINE_H__
|
|
#define __SCROLL_ENGINE_H__
|
|
|
|
#include <stdbool.h>
|
|
#include "config.h"
|
|
#include "file.h"
|
|
|
|
struct viewport;
|
|
struct scrollinfo;
|
|
|
|
extern void scroll_init(void) INIT_ATTR;
|
|
|
|
extern void lcd_bidir_scroll(int threshold);
|
|
extern void lcd_scroll_speed(int speed);
|
|
extern void lcd_scroll_delay(int ms);
|
|
|
|
extern void lcd_scroll_stop(void);
|
|
extern void lcd_scroll_stop_viewport(const struct viewport *vp);
|
|
extern void lcd_scroll_stop_viewport_rect(const struct viewport *vp, int x, int y, int width, int height);
|
|
extern bool lcd_scroll_now(struct scrollinfo *scroll);
|
|
#ifdef HAVE_REMOTE_LCD
|
|
extern void lcd_remote_scroll_speed(int speed);
|
|
extern void lcd_remote_scroll_delay(int ms);
|
|
|
|
extern void lcd_remote_scroll_stop(void);
|
|
extern void lcd_remote_scroll_stop_viewport(const struct viewport *vp);
|
|
extern void lcd_remote_scroll_stop_viewport_rect(const struct viewport *vp, int x, int y, int width, int height);
|
|
extern bool lcd_remote_scroll_now(struct scrollinfo *scroll);
|
|
#endif
|
|
|
|
|
|
|
|
/* internal usage, but in multiple drivers
|
|
* larger than the normal linebuffer since it holds the line a second
|
|
* time (+3 spaces) for non-bidir scrolling */
|
|
#define SCROLL_SPACING 3
|
|
#ifdef HAVE_LCD_BITMAP
|
|
#define SCROLL_LINE_SIZE (MAX_PATH + SCROLL_SPACING + 3*LCD_WIDTH/2 + 2)
|
|
#else
|
|
#define SCROLL_LINE_SIZE (MAX_PATH + SCROLL_SPACING + 3*LCD_WIDTH + 2)
|
|
#endif
|
|
|
|
struct scrollinfo
|
|
{
|
|
struct viewport* vp;
|
|
char linebuffer[9*MAX_PATH/10];
|
|
const char *line;
|
|
/* rectangle for the line */
|
|
int x, y; /* relative to the viewort */
|
|
int width, height;
|
|
/* pixel to skip from the beginning of the string, increments as the text scrolls */
|
|
int offset;
|
|
/* scroll presently forward or backward? */
|
|
bool backward;
|
|
bool bidir;
|
|
long start_tick;
|
|
|
|
/* support for custom scrolling functions,
|
|
* must be called with ::line == NULL to indicate that the line
|
|
* stops scrolling or when the userdata pointer is going to be changed
|
|
* (the custom scroller can release the userdata then) */
|
|
void (*scroll_func)(struct scrollinfo *s);
|
|
void *userdata;
|
|
};
|
|
|
|
struct scroll_screen_info
|
|
{
|
|
struct scrollinfo * const scroll;
|
|
const int num_scroll; /* number of scrollable lines (also number of scroll structs) */
|
|
int lines; /* Number of currently scrolling lines */
|
|
long ticks; /* # of ticks between updates*/
|
|
long delay; /* ticks delay before start */
|
|
int bidir_limit; /* percent */
|
|
#ifdef HAVE_LCD_CHARCELLS
|
|
long jump_scroll_delay; /* delay between jump scroll jumps */
|
|
int jump_scroll; /* 0=off, 1=once, ..., JUMP_SCROLL_ALWAYS */
|
|
#endif
|
|
#if defined(HAVE_LCD_BITMAP) || defined(HAVE_REMOTE_LCD)
|
|
int step; /* pixels per scroll step */
|
|
#endif
|
|
#if defined(HAVE_REMOTE_LCD)
|
|
long last_scroll;
|
|
#endif
|
|
};
|
|
|
|
/** main lcd **/
|
|
#ifdef HAVE_LCD_BITMAP
|
|
#define LCD_SCROLLABLE_LINES ((LCD_HEIGHT+4)/5 < 32 ? (LCD_HEIGHT+4)/5 : 32)
|
|
#else
|
|
#define LCD_SCROLLABLE_LINES LCD_HEIGHT * 2
|
|
#endif
|
|
|
|
extern struct scroll_screen_info lcd_scroll_info;
|
|
|
|
/** remote lcd **/
|
|
#ifdef HAVE_REMOTE_LCD
|
|
#define LCD_REMOTE_SCROLLABLE_LINES \
|
|
(((LCD_REMOTE_HEIGHT+4)/5 < 32) ? (LCD_REMOTE_HEIGHT+4)/5 : 32)
|
|
extern struct scroll_screen_info lcd_remote_scroll_info;
|
|
#endif
|
|
|
|
#endif /* __SCROLL_ENGINE_H__ */
|