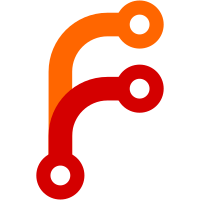
Rockbox tagcache database engine. Only host endian support at the moment and no command line parameters. Mainly for developers for debugging at the moment. git-svn-id: svn://svn.rockbox.org/rockbox/trunk@11497 a1c6a512-1295-4272-9138-f99709370657
118 lines
3.4 KiB
C
118 lines
3.4 KiB
C
/***************************************************************************
|
|
* __________ __ ___.
|
|
* Open \______ \ ____ ____ | | _\_ |__ _______ ___
|
|
* Source | _// _ \_/ ___\| |/ /| __ \ / _ \ \/ /
|
|
* Jukebox | | ( <_> ) \___| < | \_\ ( <_> > < <
|
|
* Firmware |____|_ /\____/ \___ >__|_ \|___ /\____/__/\_ \
|
|
* \/ \/ \/ \/ \/
|
|
* $Id$
|
|
*
|
|
* Copyright (C) 2005 by Miika Pekkarinen
|
|
*
|
|
* All files in this archive are subject to the GNU General Public License.
|
|
* See the file COPYING in the source tree root for full license agreement.
|
|
*
|
|
* This software is distributed on an "AS IS" basis, WITHOUT WARRANTY OF ANY
|
|
* KIND, either express or implied.
|
|
*
|
|
****************************************************************************/
|
|
#ifndef _DIRCACHE_H
|
|
#define _DIRCACHE_H
|
|
|
|
#include "dir.h"
|
|
|
|
#ifdef HAVE_DIRCACHE
|
|
|
|
#define DIRCACHE_RESERVE (1024*64)
|
|
#define DIRCACHE_LIMIT (1024*1024*6)
|
|
#define DIRCACHE_FILE ROCKBOX_DIR "/dircache.dat"
|
|
|
|
/* Internal structures. */
|
|
struct travel_data {
|
|
struct dircache_entry *first;
|
|
struct dircache_entry *ce;
|
|
struct dircache_entry *down_entry;
|
|
#ifdef SIMULATOR
|
|
DIR *dir, *newdir;
|
|
struct dirent *entry;
|
|
#else
|
|
struct fat_dir *dir;
|
|
struct fat_dir newdir;
|
|
struct fat_direntry entry;
|
|
#endif
|
|
int pathpos;
|
|
};
|
|
|
|
#define DIRCACHE_MAGIC 0x00d0c0a0
|
|
struct dircache_maindata {
|
|
long magic;
|
|
long size;
|
|
long entry_count;
|
|
struct dircache_entry *root_entry;
|
|
};
|
|
|
|
#define MAX_PENDING_BINDINGS 2
|
|
struct fdbind_queue {
|
|
char path[MAX_PATH];
|
|
int fd;
|
|
};
|
|
|
|
/* Exported structures. */
|
|
struct dircache_entry {
|
|
struct dircache_entry *next;
|
|
struct dircache_entry *up;
|
|
struct dircache_entry *down;
|
|
int attribute;
|
|
long size;
|
|
long startcluster;
|
|
unsigned short wrtdate;
|
|
unsigned short wrttime;
|
|
unsigned long name_len;
|
|
char *d_name;
|
|
};
|
|
|
|
typedef struct {
|
|
bool busy;
|
|
struct dircache_entry *entry;
|
|
struct dircache_entry *internal_entry;
|
|
struct dircache_entry secondary_entry;
|
|
DIR *regulardir;
|
|
} DIRCACHED;
|
|
|
|
void dircache_init(void);
|
|
int dircache_load(const char *path);
|
|
int dircache_save(const char *path);
|
|
int dircache_build(int last_size);
|
|
void* dircache_steal_buffer(long *size);
|
|
bool dircache_is_enabled(void);
|
|
bool dircache_is_initializing(void);
|
|
int dircache_get_entry_count(void);
|
|
int dircache_get_cache_size(void);
|
|
int dircache_get_reserve_used(void);
|
|
int dircache_get_build_ticks(void);
|
|
void dircache_disable(void);
|
|
const struct dircache_entry *dircache_get_entry_ptr(const char *filename);
|
|
void dircache_copy_path(const struct dircache_entry *entry, char *buf, int size);
|
|
|
|
void dircache_bind(int fd, const char *path);
|
|
void dircache_update_filesize(int fd, long newsize, long startcluster);
|
|
void dircache_mkdir(const char *path);
|
|
void dircache_rmdir(const char *path);
|
|
void dircache_remove(const char *name);
|
|
void dircache_rename(const char *oldpath, const char *newpath);
|
|
void dircache_add_file(const char *path, long startcluster);
|
|
|
|
DIRCACHED* opendir_cached(const char* name);
|
|
struct dircache_entry* readdir_cached(DIRCACHED* dir);
|
|
int closedir_cached(DIRCACHED *dir);
|
|
|
|
#else /* HAVE_DIRCACHE */
|
|
# define DIRCACHED DIR
|
|
# define dircache_entry dirent
|
|
# define opendir_cached opendir
|
|
# define closedir_cached closedir
|
|
# define readdir_cached readdir
|
|
# define closedir_cached closedir
|
|
#endif /* !HAVE_DIRCACHE */
|
|
|
|
#endif
|