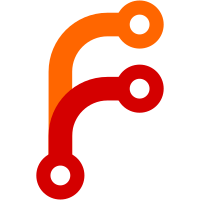
I'm currently running up against the limitations of the lcd_draw functions I want these functions to be able to be used on any size buffer not just buffers with a stride matching the underlying device [DONE] allow the framebuffer to be decoupled from the device framebuffer [DONE need examples] allow for some simple blit like transformations [DONE] remove the device framebuffer from the plugin api [DONE}ditto remote framebuffer [DONE] remove _viewport_get_framebuffer you can call struct *vp = lcd_set_viewport(NULL) and vp->buffer->fb_ptr while remote lcds may compile (and work in the sim) its not been tested on targets [FIXED] backdrops need work to be screen agnostic [FIXED] screen statusbar is not being combined into the main viewport correctly yet [FIXED] screen elements are displayed incorrectly after switch to void* [FIXED] core didn't restore proper viewport on splash etc. [NEEDS TESTING] remote lcd garbled data [FIXED] osd lib garbled screen on bmp_part [FIXED] grey_set_vp needs to return old viewport like lcd_set_viewport [FIXED] Viewport update now handles viewports with differing buffers/strides by copying to the main buffer [FIXED] splash on top of WPS leaves old framebuffer data (doesn't redraw) [UPDATE] refined this a bit more to have clear_viewport set the clean bit and have skin_render do its own screen clear scrolling viewports no longer trigger wps refresh also fixed a bug where guisyncyesno was displaying and then disappearing [ADDED!] New LCD macros that allow you to create properly size frame buffers in you desired size without wasting bytes (LCD_ and LCD_REMOTE_) LCD_STRIDE(w, h) same as STRIDE_MAIN LCD_FBSTRIDE(w, h) returns target specific stride for a buffer W x H LCD_NBELEMS(w, h) returns the number of fb_data sized elemenst needed for a buffer W x H LCD_NATIVE_STRIDE(s) conversion between rockbox native vertical and lcd native stride (2bitH) test_viewports.c has an example of usage [FIXED!!] 2bit targets don't respect non-native strides [FIXED] Few define snags Change-Id: I0d04c3834e464eca84a5a715743a297a0cefd0af
121 lines
3.7 KiB
C
121 lines
3.7 KiB
C
#include "zxvid_com.h"
|
|
|
|
#ifndef USE_GREY
|
|
/* screen routines for greyscale targets not using greyscale lib */
|
|
|
|
#if LCD_PIXELFORMAT == HORIZONTAL_PACKING
|
|
#define FB_WIDTH ((LCD_WIDTH+3)/4)
|
|
fb_data pixmask[4] ICONST_ATTR = {
|
|
0xC0, 0x30, 0x0C, 0x03
|
|
};
|
|
#elif LCD_PIXELFORMAT == VERTICAL_PACKING
|
|
fb_data pixmask[4] ICONST_ATTR = {
|
|
0x03, 0x0C, 0x30, 0xC0
|
|
};
|
|
#elif LCD_PIXELFORMAT == VERTICAL_INTERLEAVED
|
|
fb_data pixmask[8] ICONST_ATTR = {
|
|
0x0101, 0x0202, 0x0404, 0x0808, 0x1010, 0x2020, 0x4040, 0x8080
|
|
};
|
|
fb_data pixval[4] ICONST_ATTR = {
|
|
0x0000, 0x0001, 0x0100, 0x0101
|
|
};
|
|
#endif
|
|
|
|
static const unsigned char graylevels[16] = {
|
|
0, 1, 1, 1, 2, 2, 3, 3,
|
|
0, 1, 1, 1, 2, 2, 3, 3
|
|
};
|
|
|
|
static fb_data *lcd_fb = NULL;
|
|
|
|
void init_spect_scr(void)
|
|
{
|
|
int i;
|
|
unsigned mask = settings.invert_colors ? 0 : 3;
|
|
|
|
for(i = 0; i < 16; i++)
|
|
sp_colors[i] = graylevels[i] ^ mask;
|
|
|
|
sp_image = (char *) &image_array;
|
|
spscr_init_mask_color();
|
|
spscr_init_line_pointers(HEIGHT);
|
|
|
|
}
|
|
void update_screen(void)
|
|
{
|
|
if (!lcd_fb)
|
|
{
|
|
struct viewport *vp_main = rb->lcd_set_viewport(NULL);
|
|
lcd_fb = vp_main->buffer->fb_ptr;
|
|
}
|
|
fb_data *frameb;
|
|
int y=0;
|
|
int x=0;
|
|
unsigned char* image;
|
|
int srcx, srcy=0; /* x / y coordinates in source image */
|
|
image = sp_image + ( (Y_OFF)*(WIDTH) ) + X_OFF;
|
|
unsigned mask;
|
|
#if LCD_PIXELFORMAT == HORIZONTAL_PACKING
|
|
for(y = 0; y < LCD_HEIGHT; y++)
|
|
{
|
|
frameb = lcd_fb + (y) * FB_WIDTH;
|
|
srcx = 0; /* reset our x counter before each row... */
|
|
for(x = 0; x < LCD_WIDTH; x++)
|
|
{
|
|
mask = ~pixmask[x & 3];
|
|
frameb[x >> 2] = (frameb[x >> 2] & mask) | ((image[(srcx>>16)]&0x3) << ((3-(x & 3 )) * 2 ));
|
|
srcx += X_STEP; /* move through source image */
|
|
}
|
|
srcy += Y_STEP; /* move through the source image... */
|
|
image += (srcy>>16)*WIDTH; /* and possibly to the next row. */
|
|
srcy &= 0xffff; /* set up the y-coordinate between 0 and 1 */
|
|
}
|
|
#elif LCD_PIXELFORMAT == VERTICAL_PACKING
|
|
int shift;
|
|
for(y = 0; y < LCD_HEIGHT; y++)
|
|
{
|
|
frameb = lcd_fb + (y/4) * LCD_WIDTH;
|
|
srcx = 0; /* reset our x counter before each row... */
|
|
shift = ((y & 3 ) * 2 );
|
|
mask = ~pixmask[y & 3];
|
|
for(x = 0; x < LCD_WIDTH; x++)
|
|
{
|
|
frameb[x] = (frameb[x] & mask) | ((image[(srcx>>16)]&0x3) << shift );
|
|
srcx += X_STEP; /* move through source image */
|
|
}
|
|
srcy += Y_STEP; /* move through the source image... */
|
|
image += (srcy>>16)*WIDTH; /* and possibly to the next row. */
|
|
srcy &= 0xffff; /* set up the y-coordinate between 0 and 1 */
|
|
}
|
|
#elif LCD_PIXELFORMAT == VERTICAL_INTERLEAVED
|
|
int shift;
|
|
for(y = 0; y < LCD_HEIGHT; y++)
|
|
{
|
|
frameb = lcd_fb + (y/8) * LCD_WIDTH;
|
|
srcx = 0; /* reset our x counter before each row... */
|
|
shift = (y & 7);
|
|
mask = ~pixmask[y & 7];
|
|
for(x = 0; x < LCD_WIDTH; x++)
|
|
{
|
|
frameb[x] = (frameb[x] & mask) | (pixval[image[(srcx>>16)]&0x3] << shift );
|
|
srcx += X_STEP; /* move through source image */
|
|
}
|
|
srcy += Y_STEP; /* move through the source image... */
|
|
image += (srcy>>16)*WIDTH; /* and possibly to the next row. */
|
|
srcy &= 0xffff; /* set up the y-coordinate between 0 and 1 */
|
|
}
|
|
#endif
|
|
|
|
if ( settings.showfps ) {
|
|
int percent=0;
|
|
int TPF = HZ/50;/* ticks per frame */
|
|
if ((*rb->current_tick-start_time) > TPF )
|
|
percent = 100*video_frames/((*rb->current_tick-start_time)/TPF);
|
|
rb->lcd_putsxyf(0,0,"%d %%",percent);
|
|
}
|
|
|
|
|
|
rb -> lcd_update();
|
|
}
|
|
|
|
#endif /* !USE_GREY */
|