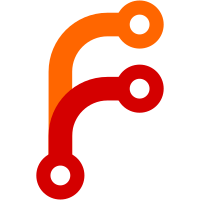
Don't build str(n)casecmp as functions if they are already defined by preprocessor git-svn-id: svn://svn.rockbox.org/rockbox/trunk@31147 a1c6a512-1295-4272-9138-f99709370657
32 lines
581 B
C
32 lines
581 B
C
|
|
#include <string.h>
|
|
#include <ctype.h>
|
|
|
|
#ifndef strcasecmp
|
|
int strcasecmp(const char *s1, const char *s2)
|
|
{
|
|
while (*s1 != '\0' && tolower(*s1) == tolower(*s2)) {
|
|
s1++;
|
|
s2++;
|
|
}
|
|
|
|
return tolower(*(unsigned char *) s1) - tolower(*(unsigned char *) s2);
|
|
}
|
|
#endif
|
|
|
|
#ifndef strncasecmp
|
|
int strncasecmp(const char *s1, const char *s2, size_t n)
|
|
{
|
|
int d = 0;
|
|
|
|
for(; n != 0; n--)
|
|
{
|
|
int c1 = tolower(*s1++);
|
|
int c2 = tolower(*s2++);
|
|
if((d = c1 - c2) != 0 || c2 == '\0')
|
|
break;
|
|
}
|
|
|
|
return d;
|
|
}
|
|
#endif
|