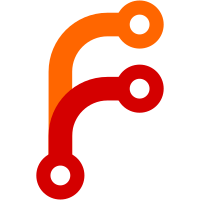
later. We still need to hunt down snippets used that are not. 1324 modified files... http://www.rockbox.org/mail/archive/rockbox-dev-archive-2008-06/0060.shtml git-svn-id: svn://svn.rockbox.org/rockbox/trunk@17847 a1c6a512-1295-4272-9138-f99709370657
128 lines
4.4 KiB
C
128 lines
4.4 KiB
C
/***************************************************************************
|
|
* __________ __ ___.
|
|
* Open \______ \ ____ ____ | | _\_ |__ _______ ___
|
|
* Source | _// _ \_/ ___\| |/ /| __ \ / _ \ \/ /
|
|
* Jukebox | | ( <_> ) \___| < | \_\ ( <_> > < <
|
|
* Firmware |____|_ /\____/ \___ >__|_ \|___ /\____/__/\_ \
|
|
* \/ \/ \/ \/ \/
|
|
* $Id$
|
|
*
|
|
* Copyright (c) 2002 by Greg Haerr <greg@censoft.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This software is distributed on an "AS IS" basis, WITHOUT WARRANTY OF ANY
|
|
* KIND, either express or implied.
|
|
*
|
|
****************************************************************************/
|
|
#ifndef _FONT_H
|
|
#define _FONT_H
|
|
|
|
#include "inttypes.h"
|
|
|
|
/*
|
|
* Incore font and image definitions
|
|
*/
|
|
#include "config.h"
|
|
|
|
#if defined(HAVE_LCD_BITMAP) || defined(SIMULATOR)
|
|
#ifndef __PCTOOL__
|
|
#include "sysfont.h"
|
|
#endif
|
|
|
|
/* max static loadable font buffer size */
|
|
#ifndef MAX_FONT_SIZE
|
|
#if LCD_HEIGHT > 64
|
|
#if MEM > 2
|
|
#define MAX_FONT_SIZE 60000
|
|
#else
|
|
#define MAX_FONT_SIZE 10000
|
|
#endif
|
|
#else
|
|
#define MAX_FONT_SIZE 4000
|
|
#endif
|
|
#endif
|
|
|
|
#ifndef FONT_HEADER_SIZE
|
|
#define FONT_HEADER_SIZE 36
|
|
#endif
|
|
|
|
#define GLYPH_CACHE_FILE "/.rockbox/.glyphcache"
|
|
|
|
/*
|
|
* Fonts are specified by number, and used for display
|
|
* of menu information as well as mp3 filename data.
|
|
* At system startup, up to MAXFONTS fonts are initialized,
|
|
* either by being compiled-in, or loaded from disk.
|
|
* If the font asked for does not exist, then the
|
|
* system uses the next lower font number. Font 0
|
|
* must be available at system startup.
|
|
* Fonts are specified in firmware/font.c.
|
|
*/
|
|
enum {
|
|
FONT_SYSFIXED, /* system fixed pitch font*/
|
|
FONT_UI, /* system porportional font*/
|
|
MAXFONTS
|
|
};
|
|
|
|
/*
|
|
* .fnt loadable font file format definition
|
|
*
|
|
* format len description
|
|
* ------------------------- ---- ------------------------------
|
|
* UCHAR version[4] 4 magic number and version bytes
|
|
* USHORT maxwidth 2 font max width in pixels
|
|
* USHORT height 2 font height in pixels
|
|
* USHORT ascent 2 font ascent (baseline) in pixels
|
|
* USHORT pad 2 unused, pad to 32-bit boundary
|
|
* ULONG firstchar 4 first character code in font
|
|
* ULONG defaultchar 4 default character code in font
|
|
* ULONG size 4 # characters in font
|
|
* ULONG nbits 4 # bytes imagebits data in file
|
|
* ULONG noffset 4 # longs offset data in file
|
|
* ULONG nwidth 4 # bytes width data in file
|
|
* MWIMAGEBITS bits nbits image bits variable data
|
|
* [MWIMAGEBITS padded to 16-bit boundary]
|
|
* USHORT offset noffset*2 offset variable data
|
|
* UCHAR width nwidth*1 width variable data
|
|
*/
|
|
|
|
/* loadable font magic and version #*/
|
|
#define VERSION "RB12"
|
|
|
|
/* builtin C-based proportional/fixed font structure */
|
|
/* based on The Microwindows Project http://microwindows.org */
|
|
struct font {
|
|
int maxwidth; /* max width in pixels*/
|
|
unsigned int height; /* height in pixels*/
|
|
int ascent; /* ascent (baseline) height*/
|
|
int firstchar; /* first character in bitmap*/
|
|
int size; /* font size in glyphs*/
|
|
const unsigned char *bits; /* 8-bit column bitmap data*/
|
|
const unsigned short *offset; /* offsets into bitmap data*/
|
|
const unsigned char *width; /* character widths or NULL if fixed*/
|
|
int defaultchar; /* default char (not glyph index)*/
|
|
int32_t bits_size; /* # bytes of glyph bits*/
|
|
};
|
|
|
|
/* font routines*/
|
|
void font_init(void);
|
|
struct font* font_load(const char *path);
|
|
struct font* font_get(int font);
|
|
void font_reset(void);
|
|
int font_getstringsize(const unsigned char *str, int *w, int *h, int fontnumber);
|
|
int font_get_width(struct font* ft, unsigned short ch);
|
|
const unsigned char * font_get_bits(struct font* ft, unsigned short ch);
|
|
void glyph_cache_save(void);
|
|
|
|
#else /* HAVE_LCD_BITMAP */
|
|
|
|
#define font_init()
|
|
#define font_load(x)
|
|
|
|
#endif
|
|
|
|
#endif
|