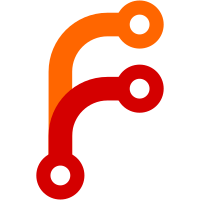
ipod2c is identical to bin2c except it skipping the header of the input files. Add this behaviour as option to bin2c to be able of using bin2c instead of ipod2c. Change-Id: I71afcaca6f2f6b0fce4c6aa3dff6be5bb205f384
174 lines
3.8 KiB
C
174 lines
3.8 KiB
C
/***************************************************************************
|
|
* __________ __ ___.
|
|
* Open \______ \ ____ ____ | | _\_ |__ _______ ___
|
|
* Source | _// _ \_/ ___\| |/ /| __ \ / _ \ \/ /
|
|
* Jukebox | | ( <_> ) \___| < | \_\ ( <_> > < <
|
|
* Firmware |____|_ /\____/ \___ >__|_ \|___ /\____/__/\_ \
|
|
* \/ \/ \/ \/ \/
|
|
*
|
|
* Copyright (C) 2007 Dave Chapman
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This software is distributed on an "AS IS" basis, WITHOUT WARRANTY OF ANY
|
|
* KIND, either express or implied.
|
|
*
|
|
****************************************************************************/
|
|
|
|
#include <stdio.h>
|
|
#include <string.h>
|
|
#include <sys/types.h>
|
|
#include <sys/stat.h>
|
|
#include <fcntl.h>
|
|
#include <stdlib.h>
|
|
#if !defined(_MSC_VER)
|
|
#include <unistd.h>
|
|
#else
|
|
#include <io.h>
|
|
#define snprintf _snprintf
|
|
#define open _open
|
|
#define close _close
|
|
#define read _read
|
|
#endif
|
|
#include <libgen.h>
|
|
|
|
#ifndef O_BINARY
|
|
#define O_BINARY 0
|
|
#endif
|
|
|
|
static void usage(void)
|
|
{
|
|
fprintf(stderr, "bin2c [options] infile cfile\n");
|
|
fprintf(stderr, " -i ipod mode\n");
|
|
}
|
|
|
|
|
|
static off_t filesize(int fd)
|
|
{
|
|
struct stat buf;
|
|
|
|
fstat(fd,&buf);
|
|
return buf.st_size;
|
|
}
|
|
|
|
static int write_cfile(const unsigned char* buf, off_t len, const char* cname)
|
|
{
|
|
char filename[256];
|
|
char filebase[256];
|
|
char* bn;
|
|
FILE* fp;
|
|
int i;
|
|
|
|
snprintf(filename,256,"%s.c",cname);
|
|
strncpy(filebase, cname, 256);
|
|
bn = basename(filebase);
|
|
|
|
fp = fopen(filename,"w+");
|
|
if (fp == NULL) {
|
|
fprintf(stderr,"Couldn't open %s\n",filename);
|
|
return -1;
|
|
}
|
|
|
|
fprintf(fp,"/* Generated by bin2c */\n\n");
|
|
fprintf(fp,"unsigned char %s[] = {",bn);
|
|
|
|
for (i=0;i<len;i++) {
|
|
if ((i % 16) == 0) {
|
|
fprintf(fp,"\n ");
|
|
}
|
|
if (i == (len-1)) {
|
|
fprintf(fp,"0x%02x",buf[i]);
|
|
} else {
|
|
fprintf(fp,"0x%02x, ",buf[i]);
|
|
}
|
|
}
|
|
fprintf(fp,"\n};\n");
|
|
|
|
fclose(fp);
|
|
return 0;
|
|
}
|
|
|
|
static int write_hfile(off_t len, const char* cname)
|
|
{
|
|
char filename[256];
|
|
char filebase[256];
|
|
char* bn;
|
|
FILE* fp;
|
|
|
|
snprintf(filename,256,"%s.h",cname);
|
|
strncpy(filebase, cname, 256);
|
|
bn = basename(filebase);
|
|
fp = fopen(filename,"w+");
|
|
if (fp == NULL) {
|
|
fprintf(stderr,"Couldn't open %s\n",filename);
|
|
return -1;
|
|
}
|
|
|
|
fprintf(fp,"/* Generated by bin2c */\n\n");
|
|
fprintf(fp,"#define LEN_%s %d\n",bn,(int)len);
|
|
fprintf(fp,"extern unsigned char %s[];\n",bn);
|
|
fclose(fp);
|
|
return 0;
|
|
}
|
|
|
|
int main (int argc, char* argv[])
|
|
{
|
|
char* infile;
|
|
char* cname;
|
|
int fd;
|
|
unsigned char* buf;
|
|
int len;
|
|
int n;
|
|
int skip = 0;
|
|
int opts = 0;
|
|
|
|
|
|
if(argc < 2) {
|
|
usage();
|
|
return 0;
|
|
}
|
|
if(strcmp(argv[1], "-i") == 0) {
|
|
skip = 8;
|
|
opts++;
|
|
}
|
|
if (argc < opts + 3) {
|
|
usage();
|
|
return 0;
|
|
}
|
|
|
|
infile=argv[opts + 1];
|
|
cname=argv[opts + 2];
|
|
|
|
fd = open(infile,O_RDONLY|O_BINARY);
|
|
if (fd < 0) {
|
|
fprintf(stderr,"Can not open %s\n",infile);
|
|
return 0;
|
|
}
|
|
|
|
len = filesize(fd) - skip;
|
|
n = lseek(fd, skip, SEEK_SET);
|
|
if (n != skip) {
|
|
fprintf(stderr,"Seek failed\n");
|
|
return 0;
|
|
}
|
|
|
|
buf = malloc(len);
|
|
n = read(fd,buf,len);
|
|
if (n < len) {
|
|
fprintf(stderr,"Short read, aborting\n");
|
|
return 0;
|
|
}
|
|
close(fd);
|
|
|
|
if (write_cfile(buf,len,cname) < 0) {
|
|
return -1;
|
|
}
|
|
if (write_hfile(len,cname) < 0) {
|
|
return -1;
|
|
}
|
|
|
|
return 0;
|
|
}
|