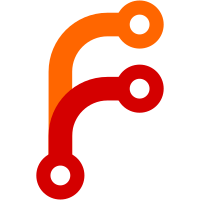
suck in the english voice strings. Thus, languages without a specified voice string for a given string where the english version has one, will get the english voice string there. git-svn-id: svn://svn.rockbox.org/rockbox/trunk@4457 a1c6a512-1295-4272-9138-f99709370657
101 lines
2.5 KiB
Perl
Executable file
101 lines
2.5 KiB
Perl
Executable file
#!/usr/bin/perl
|
|
|
|
if(!$ARGV[0]) {
|
|
print <<MOO
|
|
Usage: lang.pl <english file> <translated file>
|
|
MOO
|
|
;
|
|
exit;
|
|
}
|
|
|
|
my %ids;
|
|
open(ENG, "<$ARGV[0]");
|
|
while(<ENG>) {
|
|
if($_ =~ /^ *\#/) {
|
|
# comment
|
|
next;
|
|
}
|
|
$_ =~ s/\r//g;
|
|
if($_ =~ /^ *([a-z]+): *(.*)/) {
|
|
($var, $value) = ($1, $2);
|
|
# print "$var => $value\n";
|
|
|
|
$set{$var} = $value;
|
|
|
|
if($var eq "new") {
|
|
# the last one for a single phrase
|
|
$all{$set{'id'}, 'desc'}=$set{'desc'};
|
|
$all{$set{'id'}, 'eng'}=$set{'eng'};
|
|
$all{$set{'id'}, 'voice'}=$set{'voice'};
|
|
|
|
$ids{$set{'id'}}=1;
|
|
undef %set;
|
|
}
|
|
}
|
|
}
|
|
close(ENG);
|
|
|
|
undef %set;
|
|
open(NEW, "<$ARGV[1]");
|
|
while(<NEW>) {
|
|
if($_ =~ /^ *\#/) {
|
|
# comment
|
|
next;
|
|
}
|
|
$_ =~ s/\r//g;
|
|
|
|
if($_ =~ /^ *([a-z]+): *(.*)/) {
|
|
($var, $value) = ($1, $2);
|
|
|
|
$set{$var} = $value;
|
|
|
|
if($var eq "new") {
|
|
# the last one for a single phrase
|
|
|
|
if(!$ids{$set{'id'}}) {
|
|
print "### ".$set{'id'}." was not found in the english file!\n";
|
|
next;
|
|
}
|
|
|
|
print "\nid: ".$set{'id'}."\n";
|
|
|
|
if($set{'desc'} ne $all{$set{'id'}, 'desc'}) {
|
|
print "### Description changed! Previous description was:\n",
|
|
"### \"".$set{'desc'}."\"\n";
|
|
$set{'desc'} = $all{$set{'id'}, 'desc'};
|
|
}
|
|
print "desc: ".$set{'desc'}."\n";
|
|
|
|
if($set{'eng'} ne $all{$set{'id'}, 'eng'}) {
|
|
print "### English phrase was changed! Previous translation was made on:\n",
|
|
"### ".$set{'eng'}."\n";
|
|
$set{'eng'} = $all{$set{'id'}, 'eng'};
|
|
}
|
|
print "eng: ".$set{'eng'}."\n";
|
|
|
|
if($set{'voice'} ne $all{$set{'id'}, 'voice'}) {
|
|
print "### Voice string was changed! Previous version was:\n",
|
|
"### ".$set{'voice'}."\n";
|
|
$set{'voice'} = $all{$set{'id'}, 'voice'};
|
|
}
|
|
print "voice: ".$set{'voice'}."\n";
|
|
|
|
print "new: ".$set{'new'}."\n";
|
|
|
|
$ids{$set{'id'}}=0;
|
|
}
|
|
}
|
|
}
|
|
close(NEW);
|
|
|
|
# output new phrases not already translated
|
|
for(sort keys %ids) {
|
|
if($ids{$_}) {
|
|
my $id=$_;
|
|
print "\nid: $_\n";
|
|
print "desc: ".$all{$id, 'desc'}."\n";
|
|
print "eng: ".$all{$id, 'eng'}."\n";
|
|
print "### Not previously translated\n";
|
|
print "new: \n";
|
|
}
|
|
}
|