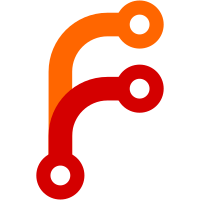
later. We still need to hunt down snippets used that are not. 1324 modified files... http://www.rockbox.org/mail/archive/rockbox-dev-archive-2008-06/0060.shtml git-svn-id: svn://svn.rockbox.org/rockbox/trunk@17847 a1c6a512-1295-4272-9138-f99709370657
127 lines
3.8 KiB
C++
127 lines
3.8 KiB
C++
/***************************************************************************
|
|
* __________ __ ___.
|
|
* Open \______ \ ____ ____ | | _\_ |__ _______ ___
|
|
* Source | _// _ \_/ ___\| |/ /| __ \ / _ \ \/ /
|
|
* Jukebox | | ( <_> ) \___| < | \_\ ( <_> > < <
|
|
* Firmware |____|_ /\____/ \___ >__|_ \|___ /\____/__/\_ \
|
|
* \/ \/ \/ \/ \/
|
|
*
|
|
* Copyright (C) 2007 by Dominik Wenger
|
|
* $Id$
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This software is distributed on an "AS IS" basis, WITHOUT WARRANTY OF ANY
|
|
* KIND, either express or implied.
|
|
*
|
|
****************************************************************************/
|
|
|
|
#ifndef INSTALLBOOTLOADER_H
|
|
#define INSTALLBOOTLOADER_H
|
|
|
|
#ifndef CONSOLE
|
|
#include <QtGui>
|
|
#else
|
|
#include <QtCore>
|
|
#endif
|
|
|
|
#include "progressloggerinterface.h"
|
|
#include "httpget.h"
|
|
#include "irivertools/irivertools.h"
|
|
|
|
#include "../ipodpatcher/ipodpatcher.h"
|
|
#include "../sansapatcher/sansapatcher.h"
|
|
|
|
bool initIpodpatcher();
|
|
bool initSansapatcher();
|
|
|
|
class BootloaderInstaller : public QObject
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
BootloaderInstaller(QObject* parent);
|
|
~BootloaderInstaller() {}
|
|
|
|
void install(ProgressloggerInterface* dp);
|
|
void uninstall(ProgressloggerInterface* dp);
|
|
|
|
void setMountPoint(QString mountpoint) {m_mountpoint = mountpoint;}
|
|
void setDevice(QString device) {m_device= device;} //!< the current plattform
|
|
void setBootloaderMethod(QString method) {m_bootloadermethod= method;}
|
|
void setBootloaderName(QString name){m_bootloadername= name;}
|
|
void setBootloaderBaseUrl(QString baseUrl){m_bootloaderUrlBase = baseUrl;}
|
|
void setOrigFirmwarePath(QString path) {m_origfirmware = path;} //!< for iriver original firmware
|
|
void setBootloaderInfoUrl(QString url) {m_bootloaderinfoUrl =url; } //!< the url for the info file
|
|
bool downloadInfo(); //!< should be called before install/uninstall, blocks until downloaded.
|
|
bool uptodate(); //!< returns wether the bootloader is uptodate
|
|
|
|
signals:
|
|
void done(bool error); //installation finished.
|
|
|
|
signals: // internal signals. Dont use this from out side.
|
|
void prepare();
|
|
void finish();
|
|
|
|
private slots:
|
|
void createInstallLog(); // adds the bootloader entry to the log
|
|
void removeInstallLog(); // removes the bootloader entry from the log
|
|
|
|
void downloadDone(bool);
|
|
void downloadRequestFinished(int, bool);
|
|
void infoDownloadDone(bool);
|
|
void infoRequestFinished(int, bool);
|
|
void installEnded(bool);
|
|
|
|
// gigabeat specific routines
|
|
void gigabeatPrepare();
|
|
void gigabeatFinish();
|
|
|
|
//iaudio specific routines
|
|
void iaudioPrepare();
|
|
void iaudioFinish();
|
|
|
|
//h10 specific routines
|
|
void h10Prepare();
|
|
void h10Finish();
|
|
|
|
//ipod specific routines
|
|
void ipodPrepare();
|
|
void ipodFinish();
|
|
|
|
//sansa specific routines
|
|
void sansaPrepare();
|
|
void sansaFinish();
|
|
|
|
//iriver specific routines
|
|
void iriverPrepare();
|
|
void iriverFinish();
|
|
|
|
//mrobe100 specific routines
|
|
void mrobe100Prepare();
|
|
void mrobe100Finish();
|
|
|
|
private:
|
|
|
|
HttpGet *infodownloader;
|
|
QTemporaryFile bootloaderInfo;
|
|
volatile bool infoDownloaded;
|
|
volatile bool infoError;
|
|
|
|
QString m_mountpoint, m_device,m_bootloadermethod,m_bootloadername;
|
|
QString m_bootloaderUrlBase,m_tempfilename,m_origfirmware;
|
|
QString m_bootloaderinfoUrl;
|
|
bool m_install;
|
|
|
|
int series,table_entry; // for fwpatcher
|
|
|
|
HttpGet *getter;
|
|
QTemporaryFile downloadFile;
|
|
|
|
ProgressloggerInterface* m_dp;
|
|
|
|
};
|
|
#endif
|