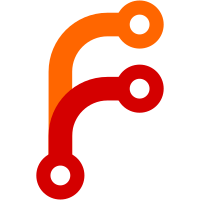
The graphical editor can now display and editor description files. The library has been improved to provide more useful function. The XML format has been slightly changed: only one soc is allowed per file (this is was already de facto the case since <soc> was the root tag). Also introduce a DTD to validate the files. Change-Id: If70ba35b6dc0242bdb87411cf4baee9597798aac
170 lines
4.6 KiB
C++
170 lines
4.6 KiB
C++
#include <QWidget>
|
|
#include <QApplication>
|
|
#include <QDesktopWidget>
|
|
#include <QFileDialog>
|
|
#include <QAction>
|
|
#include <QMenu>
|
|
#include <QMenuBar>
|
|
#include <QMessageBox>
|
|
#include <QTabBar>
|
|
#include <QCloseEvent>
|
|
#include <QDebug>
|
|
|
|
#include "mainwindow.h"
|
|
#include "regtab.h"
|
|
#include "regedit.h"
|
|
|
|
MyTabWidget::MyTabWidget()
|
|
{
|
|
setMovable(true);
|
|
setTabsClosable(true);
|
|
connect(this, SIGNAL(tabCloseRequested(int)), this, SLOT(OnCloseTab(int)));
|
|
}
|
|
|
|
bool MyTabWidget::CloseTab(int index)
|
|
{
|
|
QWidget *w = this->widget(index);
|
|
DocumentTab *doc = dynamic_cast< DocumentTab* >(w);
|
|
if(doc->Quit())
|
|
{
|
|
removeTab(index);
|
|
delete w;
|
|
return true;
|
|
}
|
|
else
|
|
return false;
|
|
}
|
|
|
|
void MyTabWidget::OnCloseTab(int index)
|
|
{
|
|
CloseTab(index);
|
|
}
|
|
|
|
MainWindow::MainWindow(Backend *backend)
|
|
:m_backend(backend)
|
|
{
|
|
QAction *new_regtab_act = new QAction(QIcon::fromTheme("document-new"), tr("Register &Tab"), this);
|
|
QAction *new_regedit_act = new QAction(QIcon::fromTheme("document-edit"), tr("Register &Editor"), this);
|
|
QAction *load_desc_act = new QAction(QIcon::fromTheme("document-open"), tr("&Soc Description"), this);
|
|
QAction *quit_act = new QAction(QIcon::fromTheme("application-exit"), tr("&Quit"), this);
|
|
QAction *about_act = new QAction(QIcon::fromTheme("help-about"), tr("&About"), this);
|
|
QAction *about_qt_act = new QAction(QIcon::fromTheme("help-about"), tr("About &Qt"), this);
|
|
|
|
connect(new_regtab_act, SIGNAL(triggered()), this, SLOT(OnNewRegTab()));
|
|
connect(new_regedit_act, SIGNAL(triggered()), this, SLOT(OnNewRegEdit()));
|
|
connect(load_desc_act, SIGNAL(triggered()), this, SLOT(OnLoadDesc()));
|
|
connect(quit_act, SIGNAL(triggered()), this, SLOT(OnQuit()));
|
|
connect(about_act, SIGNAL(triggered()), this, SLOT(OnAbout()));
|
|
connect(about_qt_act, SIGNAL(triggered()), this, SLOT(OnAboutQt()));
|
|
|
|
QMenu *file_menu = menuBar()->addMenu(tr("&File"));
|
|
QMenu *new_submenu = file_menu->addMenu(QIcon::fromTheme("document-new"), "&New");
|
|
QMenu *load_submenu = file_menu->addMenu(QIcon::fromTheme("document-open"), "&Load");
|
|
file_menu->addAction(quit_act);
|
|
|
|
new_submenu->addAction(new_regtab_act);
|
|
new_submenu->addAction(new_regedit_act);
|
|
|
|
load_submenu->addAction(load_desc_act);
|
|
|
|
QMenu *about_menu = menuBar()->addMenu(tr("&About"));
|
|
about_menu->addAction(about_act);
|
|
about_menu->addAction(about_qt_act);
|
|
|
|
m_tab = new MyTabWidget();
|
|
|
|
setCentralWidget(m_tab);
|
|
|
|
ReadSettings();
|
|
|
|
OnNewRegTab();
|
|
}
|
|
|
|
void MainWindow::ReadSettings()
|
|
{
|
|
restoreGeometry(Settings::Get()->value("mainwindow/geometry").toByteArray());
|
|
}
|
|
|
|
void MainWindow::WriteSettings()
|
|
{
|
|
Settings::Get()->setValue("mainwindow/geometry", saveGeometry());
|
|
}
|
|
|
|
void MainWindow::OnQuit()
|
|
{
|
|
close();
|
|
}
|
|
|
|
void MainWindow::OnAbout()
|
|
{
|
|
QMessageBox::about(this, "About", "Written by Amaury Pouly for Rockbox");
|
|
}
|
|
|
|
void MainWindow::OnAboutQt()
|
|
{
|
|
QMessageBox::aboutQt(this);
|
|
}
|
|
|
|
void MainWindow::closeEvent(QCloseEvent *event)
|
|
{
|
|
if(!Quit())
|
|
return event->ignore();
|
|
WriteSettings();
|
|
event->accept();
|
|
}
|
|
|
|
void MainWindow::OnLoadDesc()
|
|
{
|
|
QFileDialog *fd = new QFileDialog(this);
|
|
fd->setFilter("XML files (*.xml);;All files (*)");
|
|
fd->setFileMode(QFileDialog::ExistingFiles);
|
|
fd->setDirectory(Settings::Get()->value("loaddescdir", QDir::currentPath()).toString());
|
|
if(fd->exec())
|
|
{
|
|
QStringList filenames = fd->selectedFiles();
|
|
for(int i = 0; i < filenames.size(); i++)
|
|
if(!m_backend->LoadSocDesc(filenames[i]))
|
|
{
|
|
QMessageBox msg;
|
|
msg.setText(QString("Cannot load ") + filenames[i]);
|
|
msg.exec();
|
|
}
|
|
Settings::Get()->setValue("loaddescdir", fd->directory().absolutePath());
|
|
}
|
|
}
|
|
|
|
void MainWindow::OnTabModified(bool modified)
|
|
{
|
|
QWidget *sender = qobject_cast< QWidget* >(QObject::sender());
|
|
int index = m_tab->indexOf(sender);
|
|
if(modified)
|
|
m_tab->setTabIcon(index, QIcon::fromTheme("document-save"));
|
|
else
|
|
m_tab->setTabIcon(index, QIcon());
|
|
}
|
|
|
|
void MainWindow::AddTab(QWidget *tab, const QString& title)
|
|
{
|
|
connect(tab, SIGNAL(OnModified(bool)), this, SLOT(OnTabModified(bool)));
|
|
m_tab->setCurrentIndex(m_tab->addTab(tab, title));
|
|
}
|
|
|
|
void MainWindow::OnNewRegTab()
|
|
{
|
|
AddTab(new RegTab(m_backend, this), "Register Tab");
|
|
}
|
|
|
|
void MainWindow::OnNewRegEdit()
|
|
{
|
|
AddTab(new RegEdit(m_backend, this), "Register Editor");
|
|
}
|
|
|
|
bool MainWindow::Quit()
|
|
{
|
|
while(m_tab->count() > 0)
|
|
{
|
|
if(!m_tab->CloseTab(0))
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|