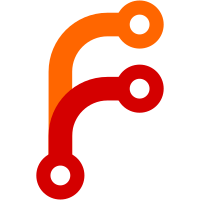
NOTE: this commit does not introduce any change, ideally even the binary should be almost the same. I checked the disassembly by hand and there are only a few differences here and there, mostly the compiler decides to compile very close expressions slightly differently. I tried to run the new code on several targets to make sure and saw no difference. The major syntax changes of the new headers are as follows: - BF_{WR,SET,CLR} are now superpowerful and allows to set several fileds at once: BF_WR(reg, field1(value1), field2(value2), ...) - BF_CS (use like BF_WR) does a write to reg_CLR and then reg_SET instead of RMW - there is no more need for macros like BF_{WR_,SET,CLR}_V, since one can simply BF_WR with field_V(name) - the old BF_SETV macro has no trivial equivalent and is replaced with its its equivalent for BF_WR(reg_SET, ...) I also rename the register headers: "regs/regs-x.h" -> "regs/x.h" to avoid the redundant "regs". Final note: the registers were generated using the following command: ./headergen_v2 -g imx -o ../../firmware/target/arm/imx233/regs/ desc/regs-stmp3{600,700,780}.xml Change-Id: I7485e8b4315a0929a8edb63e7fa1edcaa54b1edc
385 lines
22 KiB
C
385 lines
22 KiB
C
/***************************************************************************
|
|
* __________ __ ___.
|
|
* Open \______ \ ____ ____ | | _\_ |__ _______ ___
|
|
* Source | _// _ \_/ ___\| |/ /| __ \ / _ \ \/ /
|
|
* Jukebox | | ( <_> ) \___| < | \_\ ( <_> > < <
|
|
* Firmware |____|_ /\____/ \___ >__|_ \|___ /\____/__/\_ \
|
|
* \/ \/ \/ \/ \/
|
|
* This file was automatically generated by headergen, DO NOT EDIT it.
|
|
* headergen version: 3.0.0
|
|
* stmp3700 version: 2.4.0
|
|
* stmp3700 authors: Amaury Pouly
|
|
*
|
|
* Copyright (C) 2015 by the authors
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This software is distributed on an "AS IS" basis, WITHOUT WARRANTY OF ANY
|
|
* KIND, either express or implied.
|
|
*
|
|
****************************************************************************/
|
|
#ifndef __HEADERGEN_STMP3700_OCOTP_H__
|
|
#define __HEADERGEN_STMP3700_OCOTP_H__
|
|
|
|
#define HW_OCOTP_CTRL HW(OCOTP_CTRL)
|
|
#define HWA_OCOTP_CTRL (0x8002c000 + 0x0)
|
|
#define HWT_OCOTP_CTRL HWIO_32_RW
|
|
#define HWN_OCOTP_CTRL OCOTP_CTRL
|
|
#define HWI_OCOTP_CTRL
|
|
#define HW_OCOTP_CTRL_SET HW(OCOTP_CTRL_SET)
|
|
#define HWA_OCOTP_CTRL_SET (HWA_OCOTP_CTRL + 0x4)
|
|
#define HWT_OCOTP_CTRL_SET HWIO_32_WO
|
|
#define HWN_OCOTP_CTRL_SET OCOTP_CTRL
|
|
#define HWI_OCOTP_CTRL_SET
|
|
#define HW_OCOTP_CTRL_CLR HW(OCOTP_CTRL_CLR)
|
|
#define HWA_OCOTP_CTRL_CLR (HWA_OCOTP_CTRL + 0x8)
|
|
#define HWT_OCOTP_CTRL_CLR HWIO_32_WO
|
|
#define HWN_OCOTP_CTRL_CLR OCOTP_CTRL
|
|
#define HWI_OCOTP_CTRL_CLR
|
|
#define HW_OCOTP_CTRL_TOG HW(OCOTP_CTRL_TOG)
|
|
#define HWA_OCOTP_CTRL_TOG (HWA_OCOTP_CTRL + 0xc)
|
|
#define HWT_OCOTP_CTRL_TOG HWIO_32_WO
|
|
#define HWN_OCOTP_CTRL_TOG OCOTP_CTRL
|
|
#define HWI_OCOTP_CTRL_TOG
|
|
#define BP_OCOTP_CTRL_WR_UNLOCK 16
|
|
#define BM_OCOTP_CTRL_WR_UNLOCK 0xffff0000
|
|
#define BV_OCOTP_CTRL_WR_UNLOCK__KEY 0x3e77
|
|
#define BF_OCOTP_CTRL_WR_UNLOCK(v) (((v) & 0xffff) << 16)
|
|
#define BFM_OCOTP_CTRL_WR_UNLOCK(v) BM_OCOTP_CTRL_WR_UNLOCK
|
|
#define BF_OCOTP_CTRL_WR_UNLOCK_V(e) BF_OCOTP_CTRL_WR_UNLOCK(BV_OCOTP_CTRL_WR_UNLOCK__##e)
|
|
#define BFM_OCOTP_CTRL_WR_UNLOCK_V(v) BM_OCOTP_CTRL_WR_UNLOCK
|
|
#define BP_OCOTP_CTRL_RELOAD_SHADOWS 13
|
|
#define BM_OCOTP_CTRL_RELOAD_SHADOWS 0x2000
|
|
#define BF_OCOTP_CTRL_RELOAD_SHADOWS(v) (((v) & 0x1) << 13)
|
|
#define BFM_OCOTP_CTRL_RELOAD_SHADOWS(v) BM_OCOTP_CTRL_RELOAD_SHADOWS
|
|
#define BF_OCOTP_CTRL_RELOAD_SHADOWS_V(e) BF_OCOTP_CTRL_RELOAD_SHADOWS(BV_OCOTP_CTRL_RELOAD_SHADOWS__##e)
|
|
#define BFM_OCOTP_CTRL_RELOAD_SHADOWS_V(v) BM_OCOTP_CTRL_RELOAD_SHADOWS
|
|
#define BP_OCOTP_CTRL_RD_BANK_OPEN 12
|
|
#define BM_OCOTP_CTRL_RD_BANK_OPEN 0x1000
|
|
#define BF_OCOTP_CTRL_RD_BANK_OPEN(v) (((v) & 0x1) << 12)
|
|
#define BFM_OCOTP_CTRL_RD_BANK_OPEN(v) BM_OCOTP_CTRL_RD_BANK_OPEN
|
|
#define BF_OCOTP_CTRL_RD_BANK_OPEN_V(e) BF_OCOTP_CTRL_RD_BANK_OPEN(BV_OCOTP_CTRL_RD_BANK_OPEN__##e)
|
|
#define BFM_OCOTP_CTRL_RD_BANK_OPEN_V(v) BM_OCOTP_CTRL_RD_BANK_OPEN
|
|
#define BP_OCOTP_CTRL_ERROR 9
|
|
#define BM_OCOTP_CTRL_ERROR 0x200
|
|
#define BF_OCOTP_CTRL_ERROR(v) (((v) & 0x1) << 9)
|
|
#define BFM_OCOTP_CTRL_ERROR(v) BM_OCOTP_CTRL_ERROR
|
|
#define BF_OCOTP_CTRL_ERROR_V(e) BF_OCOTP_CTRL_ERROR(BV_OCOTP_CTRL_ERROR__##e)
|
|
#define BFM_OCOTP_CTRL_ERROR_V(v) BM_OCOTP_CTRL_ERROR
|
|
#define BP_OCOTP_CTRL_BUSY 8
|
|
#define BM_OCOTP_CTRL_BUSY 0x100
|
|
#define BF_OCOTP_CTRL_BUSY(v) (((v) & 0x1) << 8)
|
|
#define BFM_OCOTP_CTRL_BUSY(v) BM_OCOTP_CTRL_BUSY
|
|
#define BF_OCOTP_CTRL_BUSY_V(e) BF_OCOTP_CTRL_BUSY(BV_OCOTP_CTRL_BUSY__##e)
|
|
#define BFM_OCOTP_CTRL_BUSY_V(v) BM_OCOTP_CTRL_BUSY
|
|
#define BP_OCOTP_CTRL_ADDR 0
|
|
#define BM_OCOTP_CTRL_ADDR 0x1f
|
|
#define BF_OCOTP_CTRL_ADDR(v) (((v) & 0x1f) << 0)
|
|
#define BFM_OCOTP_CTRL_ADDR(v) BM_OCOTP_CTRL_ADDR
|
|
#define BF_OCOTP_CTRL_ADDR_V(e) BF_OCOTP_CTRL_ADDR(BV_OCOTP_CTRL_ADDR__##e)
|
|
#define BFM_OCOTP_CTRL_ADDR_V(v) BM_OCOTP_CTRL_ADDR
|
|
|
|
#define HW_OCOTP_DATA HW(OCOTP_DATA)
|
|
#define HWA_OCOTP_DATA (0x8002c000 + 0x10)
|
|
#define HWT_OCOTP_DATA HWIO_32_RW
|
|
#define HWN_OCOTP_DATA OCOTP_DATA
|
|
#define HWI_OCOTP_DATA
|
|
#define BP_OCOTP_DATA_DATA 0
|
|
#define BM_OCOTP_DATA_DATA 0xffffffff
|
|
#define BF_OCOTP_DATA_DATA(v) (((v) & 0xffffffff) << 0)
|
|
#define BFM_OCOTP_DATA_DATA(v) BM_OCOTP_DATA_DATA
|
|
#define BF_OCOTP_DATA_DATA_V(e) BF_OCOTP_DATA_DATA(BV_OCOTP_DATA_DATA__##e)
|
|
#define BFM_OCOTP_DATA_DATA_V(v) BM_OCOTP_DATA_DATA
|
|
|
|
#define HW_OCOTP_CUSTn(_n1) HW(OCOTP_CUSTn(_n1))
|
|
#define HWA_OCOTP_CUSTn(_n1) (0x8002c000 + 0x20 + (_n1) * 0x10)
|
|
#define HWT_OCOTP_CUSTn(_n1) HWIO_32_RW
|
|
#define HWN_OCOTP_CUSTn(_n1) OCOTP_CUSTn
|
|
#define HWI_OCOTP_CUSTn(_n1) (_n1)
|
|
#define BP_OCOTP_CUSTn_BITS 0
|
|
#define BM_OCOTP_CUSTn_BITS 0xffffffff
|
|
#define BF_OCOTP_CUSTn_BITS(v) (((v) & 0xffffffff) << 0)
|
|
#define BFM_OCOTP_CUSTn_BITS(v) BM_OCOTP_CUSTn_BITS
|
|
#define BF_OCOTP_CUSTn_BITS_V(e) BF_OCOTP_CUSTn_BITS(BV_OCOTP_CUSTn_BITS__##e)
|
|
#define BFM_OCOTP_CUSTn_BITS_V(v) BM_OCOTP_CUSTn_BITS
|
|
|
|
#define HW_OCOTP_CRYPTOn(_n1) HW(OCOTP_CRYPTOn(_n1))
|
|
#define HWA_OCOTP_CRYPTOn(_n1) (0x8002c000 + 0x60 + (_n1) * 0x10)
|
|
#define HWT_OCOTP_CRYPTOn(_n1) HWIO_32_RW
|
|
#define HWN_OCOTP_CRYPTOn(_n1) OCOTP_CRYPTOn
|
|
#define HWI_OCOTP_CRYPTOn(_n1) (_n1)
|
|
#define BP_OCOTP_CRYPTOn_BITS 0
|
|
#define BM_OCOTP_CRYPTOn_BITS 0xffffffff
|
|
#define BF_OCOTP_CRYPTOn_BITS(v) (((v) & 0xffffffff) << 0)
|
|
#define BFM_OCOTP_CRYPTOn_BITS(v) BM_OCOTP_CRYPTOn_BITS
|
|
#define BF_OCOTP_CRYPTOn_BITS_V(e) BF_OCOTP_CRYPTOn_BITS(BV_OCOTP_CRYPTOn_BITS__##e)
|
|
#define BFM_OCOTP_CRYPTOn_BITS_V(v) BM_OCOTP_CRYPTOn_BITS
|
|
|
|
#define HW_OCOTP_HWCAPn(_n1) HW(OCOTP_HWCAPn(_n1))
|
|
#define HWA_OCOTP_HWCAPn(_n1) (0x8002c000 + 0xa0 + (_n1) * 0x10)
|
|
#define HWT_OCOTP_HWCAPn(_n1) HWIO_32_RW
|
|
#define HWN_OCOTP_HWCAPn(_n1) OCOTP_HWCAPn
|
|
#define HWI_OCOTP_HWCAPn(_n1) (_n1)
|
|
#define BP_OCOTP_HWCAPn_BITS 0
|
|
#define BM_OCOTP_HWCAPn_BITS 0xffffffff
|
|
#define BF_OCOTP_HWCAPn_BITS(v) (((v) & 0xffffffff) << 0)
|
|
#define BFM_OCOTP_HWCAPn_BITS(v) BM_OCOTP_HWCAPn_BITS
|
|
#define BF_OCOTP_HWCAPn_BITS_V(e) BF_OCOTP_HWCAPn_BITS(BV_OCOTP_HWCAPn_BITS__##e)
|
|
#define BFM_OCOTP_HWCAPn_BITS_V(v) BM_OCOTP_HWCAPn_BITS
|
|
|
|
#define HW_OCOTP_SWCAP HW(OCOTP_SWCAP)
|
|
#define HWA_OCOTP_SWCAP (0x8002c000 + 0x100)
|
|
#define HWT_OCOTP_SWCAP HWIO_32_RW
|
|
#define HWN_OCOTP_SWCAP OCOTP_SWCAP
|
|
#define HWI_OCOTP_SWCAP
|
|
#define BP_OCOTP_SWCAP_BITS 0
|
|
#define BM_OCOTP_SWCAP_BITS 0xffffffff
|
|
#define BF_OCOTP_SWCAP_BITS(v) (((v) & 0xffffffff) << 0)
|
|
#define BFM_OCOTP_SWCAP_BITS(v) BM_OCOTP_SWCAP_BITS
|
|
#define BF_OCOTP_SWCAP_BITS_V(e) BF_OCOTP_SWCAP_BITS(BV_OCOTP_SWCAP_BITS__##e)
|
|
#define BFM_OCOTP_SWCAP_BITS_V(v) BM_OCOTP_SWCAP_BITS
|
|
|
|
#define HW_OCOTP_CUSTCAP HW(OCOTP_CUSTCAP)
|
|
#define HWA_OCOTP_CUSTCAP (0x8002c000 + 0x110)
|
|
#define HWT_OCOTP_CUSTCAP HWIO_32_RW
|
|
#define HWN_OCOTP_CUSTCAP OCOTP_CUSTCAP
|
|
#define HWI_OCOTP_CUSTCAP
|
|
#define BP_OCOTP_CUSTCAP_BITS 0
|
|
#define BM_OCOTP_CUSTCAP_BITS 0xffffffff
|
|
#define BF_OCOTP_CUSTCAP_BITS(v) (((v) & 0xffffffff) << 0)
|
|
#define BFM_OCOTP_CUSTCAP_BITS(v) BM_OCOTP_CUSTCAP_BITS
|
|
#define BF_OCOTP_CUSTCAP_BITS_V(e) BF_OCOTP_CUSTCAP_BITS(BV_OCOTP_CUSTCAP_BITS__##e)
|
|
#define BFM_OCOTP_CUSTCAP_BITS_V(v) BM_OCOTP_CUSTCAP_BITS
|
|
|
|
#define HW_OCOTP_LOCK HW(OCOTP_LOCK)
|
|
#define HWA_OCOTP_LOCK (0x8002c000 + 0x120)
|
|
#define HWT_OCOTP_LOCK HWIO_32_RW
|
|
#define HWN_OCOTP_LOCK OCOTP_LOCK
|
|
#define HWI_OCOTP_LOCK
|
|
#define BP_OCOTP_LOCK_ROM7 31
|
|
#define BM_OCOTP_LOCK_ROM7 0x80000000
|
|
#define BF_OCOTP_LOCK_ROM7(v) (((v) & 0x1) << 31)
|
|
#define BFM_OCOTP_LOCK_ROM7(v) BM_OCOTP_LOCK_ROM7
|
|
#define BF_OCOTP_LOCK_ROM7_V(e) BF_OCOTP_LOCK_ROM7(BV_OCOTP_LOCK_ROM7__##e)
|
|
#define BFM_OCOTP_LOCK_ROM7_V(v) BM_OCOTP_LOCK_ROM7
|
|
#define BP_OCOTP_LOCK_ROM6 30
|
|
#define BM_OCOTP_LOCK_ROM6 0x40000000
|
|
#define BF_OCOTP_LOCK_ROM6(v) (((v) & 0x1) << 30)
|
|
#define BFM_OCOTP_LOCK_ROM6(v) BM_OCOTP_LOCK_ROM6
|
|
#define BF_OCOTP_LOCK_ROM6_V(e) BF_OCOTP_LOCK_ROM6(BV_OCOTP_LOCK_ROM6__##e)
|
|
#define BFM_OCOTP_LOCK_ROM6_V(v) BM_OCOTP_LOCK_ROM6
|
|
#define BP_OCOTP_LOCK_ROM5 29
|
|
#define BM_OCOTP_LOCK_ROM5 0x20000000
|
|
#define BF_OCOTP_LOCK_ROM5(v) (((v) & 0x1) << 29)
|
|
#define BFM_OCOTP_LOCK_ROM5(v) BM_OCOTP_LOCK_ROM5
|
|
#define BF_OCOTP_LOCK_ROM5_V(e) BF_OCOTP_LOCK_ROM5(BV_OCOTP_LOCK_ROM5__##e)
|
|
#define BFM_OCOTP_LOCK_ROM5_V(v) BM_OCOTP_LOCK_ROM5
|
|
#define BP_OCOTP_LOCK_ROM4 28
|
|
#define BM_OCOTP_LOCK_ROM4 0x10000000
|
|
#define BF_OCOTP_LOCK_ROM4(v) (((v) & 0x1) << 28)
|
|
#define BFM_OCOTP_LOCK_ROM4(v) BM_OCOTP_LOCK_ROM4
|
|
#define BF_OCOTP_LOCK_ROM4_V(e) BF_OCOTP_LOCK_ROM4(BV_OCOTP_LOCK_ROM4__##e)
|
|
#define BFM_OCOTP_LOCK_ROM4_V(v) BM_OCOTP_LOCK_ROM4
|
|
#define BP_OCOTP_LOCK_ROM3 27
|
|
#define BM_OCOTP_LOCK_ROM3 0x8000000
|
|
#define BF_OCOTP_LOCK_ROM3(v) (((v) & 0x1) << 27)
|
|
#define BFM_OCOTP_LOCK_ROM3(v) BM_OCOTP_LOCK_ROM3
|
|
#define BF_OCOTP_LOCK_ROM3_V(e) BF_OCOTP_LOCK_ROM3(BV_OCOTP_LOCK_ROM3__##e)
|
|
#define BFM_OCOTP_LOCK_ROM3_V(v) BM_OCOTP_LOCK_ROM3
|
|
#define BP_OCOTP_LOCK_ROM2 26
|
|
#define BM_OCOTP_LOCK_ROM2 0x4000000
|
|
#define BF_OCOTP_LOCK_ROM2(v) (((v) & 0x1) << 26)
|
|
#define BFM_OCOTP_LOCK_ROM2(v) BM_OCOTP_LOCK_ROM2
|
|
#define BF_OCOTP_LOCK_ROM2_V(e) BF_OCOTP_LOCK_ROM2(BV_OCOTP_LOCK_ROM2__##e)
|
|
#define BFM_OCOTP_LOCK_ROM2_V(v) BM_OCOTP_LOCK_ROM2
|
|
#define BP_OCOTP_LOCK_ROM1 25
|
|
#define BM_OCOTP_LOCK_ROM1 0x2000000
|
|
#define BF_OCOTP_LOCK_ROM1(v) (((v) & 0x1) << 25)
|
|
#define BFM_OCOTP_LOCK_ROM1(v) BM_OCOTP_LOCK_ROM1
|
|
#define BF_OCOTP_LOCK_ROM1_V(e) BF_OCOTP_LOCK_ROM1(BV_OCOTP_LOCK_ROM1__##e)
|
|
#define BFM_OCOTP_LOCK_ROM1_V(v) BM_OCOTP_LOCK_ROM1
|
|
#define BP_OCOTP_LOCK_ROM0 24
|
|
#define BM_OCOTP_LOCK_ROM0 0x1000000
|
|
#define BF_OCOTP_LOCK_ROM0(v) (((v) & 0x1) << 24)
|
|
#define BFM_OCOTP_LOCK_ROM0(v) BM_OCOTP_LOCK_ROM0
|
|
#define BF_OCOTP_LOCK_ROM0_V(e) BF_OCOTP_LOCK_ROM0(BV_OCOTP_LOCK_ROM0__##e)
|
|
#define BFM_OCOTP_LOCK_ROM0_V(v) BM_OCOTP_LOCK_ROM0
|
|
#define BP_OCOTP_LOCK_HWSW_SHADOW_ALT 23
|
|
#define BM_OCOTP_LOCK_HWSW_SHADOW_ALT 0x800000
|
|
#define BF_OCOTP_LOCK_HWSW_SHADOW_ALT(v) (((v) & 0x1) << 23)
|
|
#define BFM_OCOTP_LOCK_HWSW_SHADOW_ALT(v) BM_OCOTP_LOCK_HWSW_SHADOW_ALT
|
|
#define BF_OCOTP_LOCK_HWSW_SHADOW_ALT_V(e) BF_OCOTP_LOCK_HWSW_SHADOW_ALT(BV_OCOTP_LOCK_HWSW_SHADOW_ALT__##e)
|
|
#define BFM_OCOTP_LOCK_HWSW_SHADOW_ALT_V(v) BM_OCOTP_LOCK_HWSW_SHADOW_ALT
|
|
#define BP_OCOTP_LOCK_CRYPTODCP_ALT 22
|
|
#define BM_OCOTP_LOCK_CRYPTODCP_ALT 0x400000
|
|
#define BF_OCOTP_LOCK_CRYPTODCP_ALT(v) (((v) & 0x1) << 22)
|
|
#define BFM_OCOTP_LOCK_CRYPTODCP_ALT(v) BM_OCOTP_LOCK_CRYPTODCP_ALT
|
|
#define BF_OCOTP_LOCK_CRYPTODCP_ALT_V(e) BF_OCOTP_LOCK_CRYPTODCP_ALT(BV_OCOTP_LOCK_CRYPTODCP_ALT__##e)
|
|
#define BFM_OCOTP_LOCK_CRYPTODCP_ALT_V(v) BM_OCOTP_LOCK_CRYPTODCP_ALT
|
|
#define BP_OCOTP_LOCK_CRYPTOKEY_ALT 21
|
|
#define BM_OCOTP_LOCK_CRYPTOKEY_ALT 0x200000
|
|
#define BF_OCOTP_LOCK_CRYPTOKEY_ALT(v) (((v) & 0x1) << 21)
|
|
#define BFM_OCOTP_LOCK_CRYPTOKEY_ALT(v) BM_OCOTP_LOCK_CRYPTOKEY_ALT
|
|
#define BF_OCOTP_LOCK_CRYPTOKEY_ALT_V(e) BF_OCOTP_LOCK_CRYPTOKEY_ALT(BV_OCOTP_LOCK_CRYPTOKEY_ALT__##e)
|
|
#define BFM_OCOTP_LOCK_CRYPTOKEY_ALT_V(v) BM_OCOTP_LOCK_CRYPTOKEY_ALT
|
|
#define BP_OCOTP_LOCK_PIN 20
|
|
#define BM_OCOTP_LOCK_PIN 0x100000
|
|
#define BF_OCOTP_LOCK_PIN(v) (((v) & 0x1) << 20)
|
|
#define BFM_OCOTP_LOCK_PIN(v) BM_OCOTP_LOCK_PIN
|
|
#define BF_OCOTP_LOCK_PIN_V(e) BF_OCOTP_LOCK_PIN(BV_OCOTP_LOCK_PIN__##e)
|
|
#define BFM_OCOTP_LOCK_PIN_V(v) BM_OCOTP_LOCK_PIN
|
|
#define BP_OCOTP_LOCK_OPS 19
|
|
#define BM_OCOTP_LOCK_OPS 0x80000
|
|
#define BF_OCOTP_LOCK_OPS(v) (((v) & 0x1) << 19)
|
|
#define BFM_OCOTP_LOCK_OPS(v) BM_OCOTP_LOCK_OPS
|
|
#define BF_OCOTP_LOCK_OPS_V(e) BF_OCOTP_LOCK_OPS(BV_OCOTP_LOCK_OPS__##e)
|
|
#define BFM_OCOTP_LOCK_OPS_V(v) BM_OCOTP_LOCK_OPS
|
|
#define BP_OCOTP_LOCK_UN2 18
|
|
#define BM_OCOTP_LOCK_UN2 0x40000
|
|
#define BF_OCOTP_LOCK_UN2(v) (((v) & 0x1) << 18)
|
|
#define BFM_OCOTP_LOCK_UN2(v) BM_OCOTP_LOCK_UN2
|
|
#define BF_OCOTP_LOCK_UN2_V(e) BF_OCOTP_LOCK_UN2(BV_OCOTP_LOCK_UN2__##e)
|
|
#define BFM_OCOTP_LOCK_UN2_V(v) BM_OCOTP_LOCK_UN2
|
|
#define BP_OCOTP_LOCK_UN1 17
|
|
#define BM_OCOTP_LOCK_UN1 0x20000
|
|
#define BF_OCOTP_LOCK_UN1(v) (((v) & 0x1) << 17)
|
|
#define BFM_OCOTP_LOCK_UN1(v) BM_OCOTP_LOCK_UN1
|
|
#define BF_OCOTP_LOCK_UN1_V(e) BF_OCOTP_LOCK_UN1(BV_OCOTP_LOCK_UN1__##e)
|
|
#define BFM_OCOTP_LOCK_UN1_V(v) BM_OCOTP_LOCK_UN1
|
|
#define BP_OCOTP_LOCK_UN0 16
|
|
#define BM_OCOTP_LOCK_UN0 0x10000
|
|
#define BF_OCOTP_LOCK_UN0(v) (((v) & 0x1) << 16)
|
|
#define BFM_OCOTP_LOCK_UN0(v) BM_OCOTP_LOCK_UN0
|
|
#define BF_OCOTP_LOCK_UN0_V(e) BF_OCOTP_LOCK_UN0(BV_OCOTP_LOCK_UN0__##e)
|
|
#define BFM_OCOTP_LOCK_UN0_V(v) BM_OCOTP_LOCK_UN0
|
|
#define BP_OCOTP_LOCK_UNALLOCATED 10
|
|
#define BM_OCOTP_LOCK_UNALLOCATED 0xfc00
|
|
#define BF_OCOTP_LOCK_UNALLOCATED(v) (((v) & 0x3f) << 10)
|
|
#define BFM_OCOTP_LOCK_UNALLOCATED(v) BM_OCOTP_LOCK_UNALLOCATED
|
|
#define BF_OCOTP_LOCK_UNALLOCATED_V(e) BF_OCOTP_LOCK_UNALLOCATED(BV_OCOTP_LOCK_UNALLOCATED__##e)
|
|
#define BFM_OCOTP_LOCK_UNALLOCATED_V(v) BM_OCOTP_LOCK_UNALLOCATED
|
|
#define BP_OCOTP_LOCK_CUSTCAP 9
|
|
#define BM_OCOTP_LOCK_CUSTCAP 0x200
|
|
#define BF_OCOTP_LOCK_CUSTCAP(v) (((v) & 0x1) << 9)
|
|
#define BFM_OCOTP_LOCK_CUSTCAP(v) BM_OCOTP_LOCK_CUSTCAP
|
|
#define BF_OCOTP_LOCK_CUSTCAP_V(e) BF_OCOTP_LOCK_CUSTCAP(BV_OCOTP_LOCK_CUSTCAP__##e)
|
|
#define BFM_OCOTP_LOCK_CUSTCAP_V(v) BM_OCOTP_LOCK_CUSTCAP
|
|
#define BP_OCOTP_LOCK_HWSW 8
|
|
#define BM_OCOTP_LOCK_HWSW 0x100
|
|
#define BF_OCOTP_LOCK_HWSW(v) (((v) & 0x1) << 8)
|
|
#define BFM_OCOTP_LOCK_HWSW(v) BM_OCOTP_LOCK_HWSW
|
|
#define BF_OCOTP_LOCK_HWSW_V(e) BF_OCOTP_LOCK_HWSW(BV_OCOTP_LOCK_HWSW__##e)
|
|
#define BFM_OCOTP_LOCK_HWSW_V(v) BM_OCOTP_LOCK_HWSW
|
|
#define BP_OCOTP_LOCK_CUSTCAP_SHADOW 7
|
|
#define BM_OCOTP_LOCK_CUSTCAP_SHADOW 0x80
|
|
#define BF_OCOTP_LOCK_CUSTCAP_SHADOW(v) (((v) & 0x1) << 7)
|
|
#define BFM_OCOTP_LOCK_CUSTCAP_SHADOW(v) BM_OCOTP_LOCK_CUSTCAP_SHADOW
|
|
#define BF_OCOTP_LOCK_CUSTCAP_SHADOW_V(e) BF_OCOTP_LOCK_CUSTCAP_SHADOW(BV_OCOTP_LOCK_CUSTCAP_SHADOW__##e)
|
|
#define BFM_OCOTP_LOCK_CUSTCAP_SHADOW_V(v) BM_OCOTP_LOCK_CUSTCAP_SHADOW
|
|
#define BP_OCOTP_LOCK_HWSW_SHADOW 6
|
|
#define BM_OCOTP_LOCK_HWSW_SHADOW 0x40
|
|
#define BF_OCOTP_LOCK_HWSW_SHADOW(v) (((v) & 0x1) << 6)
|
|
#define BFM_OCOTP_LOCK_HWSW_SHADOW(v) BM_OCOTP_LOCK_HWSW_SHADOW
|
|
#define BF_OCOTP_LOCK_HWSW_SHADOW_V(e) BF_OCOTP_LOCK_HWSW_SHADOW(BV_OCOTP_LOCK_HWSW_SHADOW__##e)
|
|
#define BFM_OCOTP_LOCK_HWSW_SHADOW_V(v) BM_OCOTP_LOCK_HWSW_SHADOW
|
|
#define BP_OCOTP_LOCK_CRYPTODCP 5
|
|
#define BM_OCOTP_LOCK_CRYPTODCP 0x20
|
|
#define BF_OCOTP_LOCK_CRYPTODCP(v) (((v) & 0x1) << 5)
|
|
#define BFM_OCOTP_LOCK_CRYPTODCP(v) BM_OCOTP_LOCK_CRYPTODCP
|
|
#define BF_OCOTP_LOCK_CRYPTODCP_V(e) BF_OCOTP_LOCK_CRYPTODCP(BV_OCOTP_LOCK_CRYPTODCP__##e)
|
|
#define BFM_OCOTP_LOCK_CRYPTODCP_V(v) BM_OCOTP_LOCK_CRYPTODCP
|
|
#define BP_OCOTP_LOCK_CRYPTOKEY 4
|
|
#define BM_OCOTP_LOCK_CRYPTOKEY 0x10
|
|
#define BF_OCOTP_LOCK_CRYPTOKEY(v) (((v) & 0x1) << 4)
|
|
#define BFM_OCOTP_LOCK_CRYPTOKEY(v) BM_OCOTP_LOCK_CRYPTOKEY
|
|
#define BF_OCOTP_LOCK_CRYPTOKEY_V(e) BF_OCOTP_LOCK_CRYPTOKEY(BV_OCOTP_LOCK_CRYPTOKEY__##e)
|
|
#define BFM_OCOTP_LOCK_CRYPTOKEY_V(v) BM_OCOTP_LOCK_CRYPTOKEY
|
|
#define BP_OCOTP_LOCK_CUST3 3
|
|
#define BM_OCOTP_LOCK_CUST3 0x8
|
|
#define BF_OCOTP_LOCK_CUST3(v) (((v) & 0x1) << 3)
|
|
#define BFM_OCOTP_LOCK_CUST3(v) BM_OCOTP_LOCK_CUST3
|
|
#define BF_OCOTP_LOCK_CUST3_V(e) BF_OCOTP_LOCK_CUST3(BV_OCOTP_LOCK_CUST3__##e)
|
|
#define BFM_OCOTP_LOCK_CUST3_V(v) BM_OCOTP_LOCK_CUST3
|
|
#define BP_OCOTP_LOCK_CUST2 2
|
|
#define BM_OCOTP_LOCK_CUST2 0x4
|
|
#define BF_OCOTP_LOCK_CUST2(v) (((v) & 0x1) << 2)
|
|
#define BFM_OCOTP_LOCK_CUST2(v) BM_OCOTP_LOCK_CUST2
|
|
#define BF_OCOTP_LOCK_CUST2_V(e) BF_OCOTP_LOCK_CUST2(BV_OCOTP_LOCK_CUST2__##e)
|
|
#define BFM_OCOTP_LOCK_CUST2_V(v) BM_OCOTP_LOCK_CUST2
|
|
#define BP_OCOTP_LOCK_CUST1 1
|
|
#define BM_OCOTP_LOCK_CUST1 0x2
|
|
#define BF_OCOTP_LOCK_CUST1(v) (((v) & 0x1) << 1)
|
|
#define BFM_OCOTP_LOCK_CUST1(v) BM_OCOTP_LOCK_CUST1
|
|
#define BF_OCOTP_LOCK_CUST1_V(e) BF_OCOTP_LOCK_CUST1(BV_OCOTP_LOCK_CUST1__##e)
|
|
#define BFM_OCOTP_LOCK_CUST1_V(v) BM_OCOTP_LOCK_CUST1
|
|
#define BP_OCOTP_LOCK_CUST0 0
|
|
#define BM_OCOTP_LOCK_CUST0 0x1
|
|
#define BF_OCOTP_LOCK_CUST0(v) (((v) & 0x1) << 0)
|
|
#define BFM_OCOTP_LOCK_CUST0(v) BM_OCOTP_LOCK_CUST0
|
|
#define BF_OCOTP_LOCK_CUST0_V(e) BF_OCOTP_LOCK_CUST0(BV_OCOTP_LOCK_CUST0__##e)
|
|
#define BFM_OCOTP_LOCK_CUST0_V(v) BM_OCOTP_LOCK_CUST0
|
|
|
|
#define HW_OCOTP_OPSn(_n1) HW(OCOTP_OPSn(_n1))
|
|
#define HWA_OCOTP_OPSn(_n1) (0x8002c000 + 0x130 + (_n1) * 0x10)
|
|
#define HWT_OCOTP_OPSn(_n1) HWIO_32_RW
|
|
#define HWN_OCOTP_OPSn(_n1) OCOTP_OPSn
|
|
#define HWI_OCOTP_OPSn(_n1) (_n1)
|
|
#define BP_OCOTP_OPSn_BITS 0
|
|
#define BM_OCOTP_OPSn_BITS 0xffffffff
|
|
#define BF_OCOTP_OPSn_BITS(v) (((v) & 0xffffffff) << 0)
|
|
#define BFM_OCOTP_OPSn_BITS(v) BM_OCOTP_OPSn_BITS
|
|
#define BF_OCOTP_OPSn_BITS_V(e) BF_OCOTP_OPSn_BITS(BV_OCOTP_OPSn_BITS__##e)
|
|
#define BFM_OCOTP_OPSn_BITS_V(v) BM_OCOTP_OPSn_BITS
|
|
|
|
#define HW_OCOTP_UNn(_n1) HW(OCOTP_UNn(_n1))
|
|
#define HWA_OCOTP_UNn(_n1) (0x8002c000 + 0x170 + (_n1) * 0x10)
|
|
#define HWT_OCOTP_UNn(_n1) HWIO_32_RW
|
|
#define HWN_OCOTP_UNn(_n1) OCOTP_UNn
|
|
#define HWI_OCOTP_UNn(_n1) (_n1)
|
|
#define BP_OCOTP_UNn_BITS 0
|
|
#define BM_OCOTP_UNn_BITS 0xffffffff
|
|
#define BF_OCOTP_UNn_BITS(v) (((v) & 0xffffffff) << 0)
|
|
#define BFM_OCOTP_UNn_BITS(v) BM_OCOTP_UNn_BITS
|
|
#define BF_OCOTP_UNn_BITS_V(e) BF_OCOTP_UNn_BITS(BV_OCOTP_UNn_BITS__##e)
|
|
#define BFM_OCOTP_UNn_BITS_V(v) BM_OCOTP_UNn_BITS
|
|
|
|
#define HW_OCOTP_ROMn(_n1) HW(OCOTP_ROMn(_n1))
|
|
#define HWA_OCOTP_ROMn(_n1) (0x8002c000 + 0x1a0 + (_n1) * 0x10)
|
|
#define HWT_OCOTP_ROMn(_n1) HWIO_32_RW
|
|
#define HWN_OCOTP_ROMn(_n1) OCOTP_ROMn
|
|
#define HWI_OCOTP_ROMn(_n1) (_n1)
|
|
#define BP_OCOTP_ROMn_BITS 0
|
|
#define BM_OCOTP_ROMn_BITS 0xffffffff
|
|
#define BF_OCOTP_ROMn_BITS(v) (((v) & 0xffffffff) << 0)
|
|
#define BFM_OCOTP_ROMn_BITS(v) BM_OCOTP_ROMn_BITS
|
|
#define BF_OCOTP_ROMn_BITS_V(e) BF_OCOTP_ROMn_BITS(BV_OCOTP_ROMn_BITS__##e)
|
|
#define BFM_OCOTP_ROMn_BITS_V(v) BM_OCOTP_ROMn_BITS
|
|
|
|
#define HW_OCOTP_VERSION HW(OCOTP_VERSION)
|
|
#define HWA_OCOTP_VERSION (0x8002c000 + 0x220)
|
|
#define HWT_OCOTP_VERSION HWIO_32_RW
|
|
#define HWN_OCOTP_VERSION OCOTP_VERSION
|
|
#define HWI_OCOTP_VERSION
|
|
#define BP_OCOTP_VERSION_MAJOR 24
|
|
#define BM_OCOTP_VERSION_MAJOR 0xff000000
|
|
#define BF_OCOTP_VERSION_MAJOR(v) (((v) & 0xff) << 24)
|
|
#define BFM_OCOTP_VERSION_MAJOR(v) BM_OCOTP_VERSION_MAJOR
|
|
#define BF_OCOTP_VERSION_MAJOR_V(e) BF_OCOTP_VERSION_MAJOR(BV_OCOTP_VERSION_MAJOR__##e)
|
|
#define BFM_OCOTP_VERSION_MAJOR_V(v) BM_OCOTP_VERSION_MAJOR
|
|
#define BP_OCOTP_VERSION_MINOR 16
|
|
#define BM_OCOTP_VERSION_MINOR 0xff0000
|
|
#define BF_OCOTP_VERSION_MINOR(v) (((v) & 0xff) << 16)
|
|
#define BFM_OCOTP_VERSION_MINOR(v) BM_OCOTP_VERSION_MINOR
|
|
#define BF_OCOTP_VERSION_MINOR_V(e) BF_OCOTP_VERSION_MINOR(BV_OCOTP_VERSION_MINOR__##e)
|
|
#define BFM_OCOTP_VERSION_MINOR_V(v) BM_OCOTP_VERSION_MINOR
|
|
#define BP_OCOTP_VERSION_STEP 0
|
|
#define BM_OCOTP_VERSION_STEP 0xffff
|
|
#define BF_OCOTP_VERSION_STEP(v) (((v) & 0xffff) << 0)
|
|
#define BFM_OCOTP_VERSION_STEP(v) BM_OCOTP_VERSION_STEP
|
|
#define BF_OCOTP_VERSION_STEP_V(e) BF_OCOTP_VERSION_STEP(BV_OCOTP_VERSION_STEP__##e)
|
|
#define BFM_OCOTP_VERSION_STEP_V(v) BM_OCOTP_VERSION_STEP
|
|
|
|
#endif /* __HEADERGEN_STMP3700_OCOTP_H__*/
|