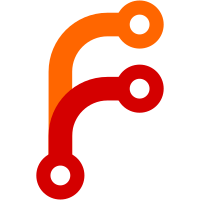
A GNU extension that returns dst + size instead of dst. It's a nice shortcut when copying strings with a known size or back-to-back blocks and you have to do it often. May of course be called directly or alternately through __builtin_mempcpy in some compiler versions. For ASM on native targets, it is implemented as an alternate entrypoint to memcpy which adds minimal code and overhead. Change-Id: I4cbb3483f6df3c1007247fe0a95fd7078737462b
47 lines
1.1 KiB
C
47 lines
1.1 KiB
C
/*
|
|
FUNCTION
|
|
<<mempcpy>>---copy memory regions and return end pointer
|
|
|
|
ANSI_SYNOPSIS
|
|
#include <string.h>
|
|
void* mempcpy(void *<[out]>, const void *<[in]>, size_t <[n]>);
|
|
|
|
TRAD_SYNOPSIS
|
|
void *mempcpy(<[out]>, <[in]>, <[n]>
|
|
void *<[out]>;
|
|
void *<[in]>;
|
|
size_t <[n]>;
|
|
|
|
DESCRIPTION
|
|
This function copies <[n]> bytes from the memory region
|
|
pointed to by <[in]> to the memory region pointed to by
|
|
<[out]>.
|
|
|
|
If the regions overlap, the behavior is undefined.
|
|
|
|
RETURNS
|
|
<<mempcpy>> returns a pointer to the byte following the
|
|
last byte copied to the <[out]> region.
|
|
|
|
PORTABILITY
|
|
<<mempcpy>> is a GNU extension.
|
|
|
|
<<mempcpy>> requires no supporting OS subroutines.
|
|
|
|
*/
|
|
|
|
#include "config.h"
|
|
#include "_ansi.h" /* for _DEFUN */
|
|
#include <string.h>
|
|
|
|
/* This may be conjoined with memcpy in <cpu>/memcpy.S to get it nearly for
|
|
free */
|
|
|
|
_PTR
|
|
_DEFUN (mempcpy, (dst0, src0, len0),
|
|
_PTR dst0 _AND
|
|
_CONST _PTR src0 _AND
|
|
size_t len0)
|
|
{
|
|
return memcpy(dst0, src0, len0) + len0;
|
|
}
|